Lumo Utility Classes
Lumo Utility Classes are predefined CSS class names and stylesheets — similar to the popular Tailwind CSS library. They can be used to style HTML elements and layouts without having to write CSS.
Each utility class applies a particular style to the element, such as background color, borders, fonts, sizing, or spacing. Classes for applying CSS flexbox and grid layout features are also available.
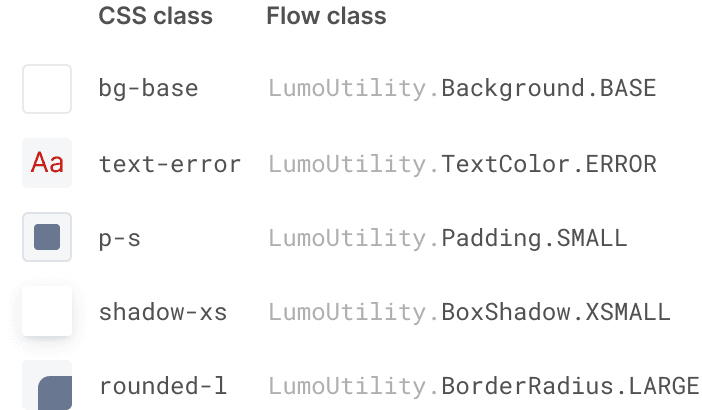
The Lumo utility classes are designed primarily to be used with native HTML elements, Vaadin layout components, and custom UI structures. Although some of them do work as expected on some Vaadin components, this is not their intended use. They especially cannot be used to style the inner parts of components.
Enable Utility Classes
The utility classes are not loaded by default. To use them, make sure that the utility
module is included in the lumoImports
list in your application theme’s configuration file:
{
"lumoImports" : [ "typography", "color", "spacing", "badge", "utility" ]
}
Vaadin application projects that are generated with start.vaadin.com already have this configured.
Using Utility Classes
Utility classes are regular CSS classes applied through CSS classnames.
The LumoUtility
Java class in Flow contains String
constants for all utility classes. They’re divided into nested category classes, such as LumoUtility.FontSize
. These are applied the same way as CSS class name literals, using the addClassNames
or ClassList
APIs.
Span errorMsg = new Span("Error");
errorMsg.addClassNames(
LumoUtility.TextColor.ERROR,
LumoUtility.Padding.SMALL,
LumoUtility.Background.BASE,
LumoUtility.BoxShadow.XSMALL,
LumoUtility.BorderRadius.LARGE
);
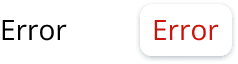
Note
|
Doesn’t Work in Embedded Overlays
Due to the way overlays (e.g., Dialog or the Combo Box drop-down) are rendered in embedded Flow UIs, the Utility Classes don’t work in them. See https://github.com/vaadin/flow/issues/16083 for details. |
List of Utility Classes
The following is a list of all of the utility classes:
Important
|
LumoUtility Java API available from version 23.1
|
Backgrounds
Classes for applying a background color.
Java class: LumoUtility.Background
Contrast
Preview | CSS Class Name / Java Constant |
---|---|
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
|
Primary
Preview | CSS Class Name / Java Constant |
---|---|
| |
| |
|
Borders
Border related classes.
Java class: LumoUtility.Border
Preview | CSS Class Name / Java Constant |
---|---|
| |
| |
| |
| |
| |
|
Border Color
Classes for setting the border color of an element.
Java class: LumoUtility.BorderColor
Contrast
Preview | CSS Class Name / Java Constant |
---|---|
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
|
Primary
Preview | CSS Class Name / Java Constant |
---|---|
| |
| |
|
Error
Preview | CSS Class Name / Java Constant |
---|---|
| |
| |
|
Box Shadow
Classes for applying a box shadow.
Java class: LumoUtility.BoxShadow
Preview | CSS Class Name / Java Constant |
---|---|
| |
| |
| |
| |
|
Sizing
Classes for setting the height and width of an element.
Height
Java class: LumoUtility.Height
Preview | CSS Class Name / Java Constant |
---|---|
N/A |
|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Auto |
|
Full |
|
Screen(scroll to see total height) |
|
Max Height
Java class: LumoUtility.MaxHeight
Preview | CSS Class Name / Java Constant |
---|---|
N/A |
|
N/A |
|
Min Height
Java class: LumoUtility.MinHeight
Preview | CSS Class Name / Java Constant |
---|---|
N/A |
|
Full |
|
Screen(scroll to see total height) |
|
Width
Java class: LumoUtility.Width
Preview | CSS Class Name / Java Constant |
---|---|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Auto |
|
Full |
|
Max Width
Java class: LumoUtility.MaxWidth
Preview | CSS Class Name / Java Constant |
---|---|
N/A |
|
N/A |
|
N/A |
|
N/A |
|
N/A |
|
N/A |
|
Spacing
Classes for applying margin and padding to individual elements, as well as spacing between elements in a layout.
Margin
Classes for setting the margin of an element.
Uniform
Java class: LumoUtility.Margin
Preview | CSS Class Name / Java Constant |
---|---|
Auto |
|
0 |
|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Bottom
Java class: LumoUtility.Margin.Bottom
Preview | CSS Class Name / Java Constant |
---|---|
Auto |
|
0 |
|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Left
Java class: LumoUtility.Margin.Left
Preview | CSS Class Name / Java Constant |
---|---|
Auto |
|
0 |
|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Right
Java class: LumoUtility.Margin.Right
Preview | CSS Class Name / Java Constant |
---|---|
Auto |
|
0 |
|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Top
Java class: LumoUtility.Margin.Top
Preview | CSS Class Name / Java Constant |
---|---|
Auto |
|
0 |
|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Inline End
Java class: LumoUtility.Margin.End
Preview | CSS Class Name / Java Constant |
---|---|
Auto |
|
0 |
|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Inline Start
Java class: LumoUtility.Margin.Start
Preview | CSS Class Name / Java Constant |
---|---|
Auto |
|
0 |
|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Padding
Classes for setting the padding of an element.
Uniform
Java class: LumoUtility.Padding
Preview | CSS Class Name / Java Constant |
---|---|
0 |
|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Bottom
Java class: LumoUtility.Padding.Bottom
Preview | CSS Class Name / Java Constant |
---|---|
0 |
|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Left
Java class: LumoUtility.Padding.Left
Preview | CSS Class Name / Java Constant |
---|---|
0 |
|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Right
Java class: LumoUtility.Padding.Right
Preview | CSS Class Name / Java Constant |
---|---|
0 |
|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Top
Java class: LumoUtility.Padding.Top
Preview | CSS Class Name / Java Constant |
---|---|
0 |
|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Inline End
Java class: LumoUtility.Padding.End
Preview | CSS Class Name / Java Constant |
---|---|
0 |
|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Inline Start
Java class: LumoUtility.Padding.Start
Preview | CSS Class Name / Java Constant |
---|---|
0 |
|
XS |
|
S |
|
M |
|
L |
|
XL |
|
Typography
Classes for styling text.
Font Size
Classes for setting the font size of an element.
Java class: LumoUtility.FontSize
Preview | CSS Class Name / Java Constant |
---|---|
Lorem ipsum |
|
Lorem ipsum |
|
Lorem ipsum |
|
Lorem ipsum |
|
Lorem ipsum |
|
Lorem ipsum |
|
Lorem ipsum |
|
Lorem ipsum |
|
Font Weight
Classes for setting the font weight of an element.
Java class: LumoUtility.FontWeight
Preview | CSS Class Name / Java Constant |
---|---|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Line Height
Classes for setting the line height of an element.
Java class: LumoUtility.LineHeight
Preview | CSS Class Name / Java Constant |
---|---|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
List Style Type
Class for remove the default look of a list.
Java class: LumoUtility.ListStyleType
Preview | CSS Class Name / Java Constant |
---|---|
|
|
Text Alignment
Classes for setting an element’s text alignment.
Java class: LumoUtility.TextAlignment
Preview | CSS Class Name / Java Constant |
---|---|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Text Color
Classes for setting the text color of an element.
Java class: LumoUtility.TextColor
Preview | CSS Class Name / Java Constant |
---|---|
Header |
|
Body |
|
Secondary |
|
Tertiary |
|
Disabled |
|
Primary |
|
Primary contrast |
|
Error |
|
Error contrast |
|
Error |
|
Error contrast |
|
Success |
|
Success contrast |
|
Text Overflow
Classes for setting text overflow.
Java class: LumoUtility.TextOverflow
Preview | CSS Class Name / Java Constant |
---|---|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. |
|
Text Transform
Classes for transforming text.
Java class: LumoUtility.TextTransform
Preview | CSS Class Name / Java Constant |
---|---|
Lorem ipsum dolor sit amet |
|
Lorem ipsum dolor sit amet |
|
Lorem ipsum dolor sit amet |
|
Whitespace
Classes for transforming text.
Java class: LumoUtility.Whitespace
Preview | CSS Class Name / Java Constant |
---|---|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. |
|
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. |
|
Flexbox & Grid
Classes for flexbox and grid layouts.
Note
|
Remember to set
The following classes require setting either the display flex or grid CSS class on the element to work. See Display classes.
|
Align Content
Classes for distributing space around and between items along a flexbox’s cross axis or a grid’s block axis. Applies to flexbox and grid layouts.
Java class: LumoUtility.AlignContent
Preview | CSS Class Name / Java Constant |
---|---|
1234 |
|
1234 |
|
1234 |
|
1234 |
|
1234 |
|
1234 |
|
1234 |
|
Align Items
Classes for aligning items along a flexbox’s cross axis or a grid’s block axis. Applies to flexbox and grid layouts.
Java class: LumoUtility.AlignItems
Preview | CSS Class Name / Java Constant |
---|---|
123 |
|
123 |
|
123 |
|
123 |
|
123 |
|
Align Self
Classes for overriding individual items align-item
property. Applies to flexbox and grid items.
Java class: LumoUtility.AlignSelf
Preview | CSS Class Name / Java Constant |
---|---|
123 |
|
123 |
|
123 |
|
123 |
|
123 |
|
123 |
|
Justify Content
Classes for aligning items along a flexbox’s main axis or a grid’s inline axis. Applies to flexbox and grid layouts.
Java class: LumoUtility.JustifyContent
Preview | CSS Class Name / Java Constant |
---|---|
12 |
|
12 |
|
12 |
|
12 |
|
12 |
|
12 |
|
Flex
Classes for setting how items grow and shrink in a flexbox layout. Applies to flexbox items.
Java class: LumoUtility.Flex
Preview | CSS Class Name / Java Constant |
---|---|
1999 |
|
1999 |
|
Flex Direction
Classes for setting the flex direction of a flexbox layout.
Java class: LumoUtility.FlexDirection
Preview | CSS Class Name / Java Constant |
---|---|
123 |
|
123 |
|
123 |
|
123 |
|
Flex Grow
Classes for setting how items grow in a flexbox layout. Applies to flexbox items.
Java class: LumoUtility.Flex
Preview | CSS Class Name / Java Constant |
---|---|
1 |
|
1 |
|
Flex Shrink
Classes for setting how items shrink in a flexbox layout. Applies to flexbox items.
Java class: LumoUtility.Flex
Preview | CSS Class Name / Java Constant |
---|---|
56px |
|
56px |
|
Flex Wrap
Classes for setting how items wrap in a flexbox layout. Applies to flexbox layouts.
Java class: LumoUtility.FlexWrap
Preview | CSS Class Name / Java Constant |
---|---|
1234 |
|
1234 |
|
1234 |
|
Gap
Classes for defining the space between items in a flexbox or grid layout. Applies to flexbox and grid layouts.
Uniform
Java class: LumoUtility.Gap
Preview | CSS Class Name / Java Constant |
---|---|
1234 |
|
1234 |
|
1234 |
|
1234 |
|
1234 |
|
Grid Columns
Classes for setting the number of columns in a grid layout.
Java class: LumoUtility.Grid.Column
Preview | CSS Class Name / Java Constant |
---|---|
1234 |
|
1234 |
|
1234 |
|
1234 |
|
12345678 |
|
12345678 |
|
12345678 |
|
12345678 |
|
123456789101112 |
|
123456789101112 |
|
123456789101112 |
|
123456789101112 |
|
Grid Rows
Classes for setting the number of rows in a grid layout.
Java class: LumoUtility.Grid.Row
Preview | CSS Class Name / Java Constant |
---|---|
12345678 |
|
12345678 |
|
12345678 |
|
12345678 |
|
12345678 |
|
12345678 |
|
Spanning Columns
Classes for setting the column span of an item in a grid layout.
Java class: LumoUtility.Grid.Column
Preview | CSS Class Name / Java Constant |
---|---|
123456789101112 |
|
1234567891011121314151617181920212223 |
|
12345678910111213141516171819202122 |
|
123456789101112131415161718192021 |
|
1234567891011121314151617181920 |
|
12345678910111213141516171819 |
|
123456789101112131415161718 |
|
1234567891011121314151617 |
|
12345678910111213141516 |
|
123456789101112131415 |
|
1234567891011121314 |
|
12345678910111213 |
|
Spanning Rows
Classes for setting the row span of an item in a grid layout.
Java class: LumoUtility.Grid.Row
Preview | CSS Class Name / Java Constant |
---|---|
123456 |
|
1234567891011 |
|
12345678910 |
|
123456789 |
|
12345678 |
|
1234567 |
|
Layout
Classes for general layout purposes.
Box Sizing
Classes for setting the box sizing property of an element. Box sizing determines whether an element’s border and padding is considered a part of its size.
Java class: LumoUtility.BoxSizing
Preview | CSS Class Name / Java Constant |
---|---|
Size: 44px |
|
Size: 44px |
|
Display
Classes for setting the display property of an element. Determines whether the element is a block or inline element and how its items are laid out.
Java class: LumoUtility.Display
Preview | CSS Class Name / Java Constant |
---|---|
123 |
|
123 |
|
123 |
|
123 |
|
123 |
|
| |
123456789 |
|
123456789 |
|
Overflow
Classes for setting the overflow behavior of an element.
Java class: LumoUtility.Overflow
Preview | CSS Class Name / Java Constant |
---|---|
123Scrolls |
|
123No scroll |
|
123Scrolls |
|
Position
Classes for setting the position of an element.
Java class: LumoUtility.Position
Preview | CSS Class Name / Java Constant |
---|---|
RelativeAbsolute |
|
FixedLorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. |
|
N/A |
|
Sticky 1Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.Sticky 2Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. |
|
N/A |
|