Connect to Java
You can connect both components and data between Java and the UI made with Designer. In practice, this is accomplished with a Java class that enables Vaadin Flow to connect your Java code to the UI. In other words, you access the UI defined in a design programmatically through the companion class for the design.
In short, to connect a component to Java make sure you have a companion Java class with @JsModule
and @Tag
annotations matching the design. Set the id attribute for the element, and click on the Connect button for the element in the Outline.
The Java Companion File
The Java companion file contains the class that connects your Java code to the UI defined in the design. There can be only one companion class for a design.
You may have already created the companion file at the time you created the design using the new design wizard. If that’s the case you are all set. You can start connecting components and data.
When the design has a companion file, the name of the companion file is displayed next to the design’s name in the toolbar.
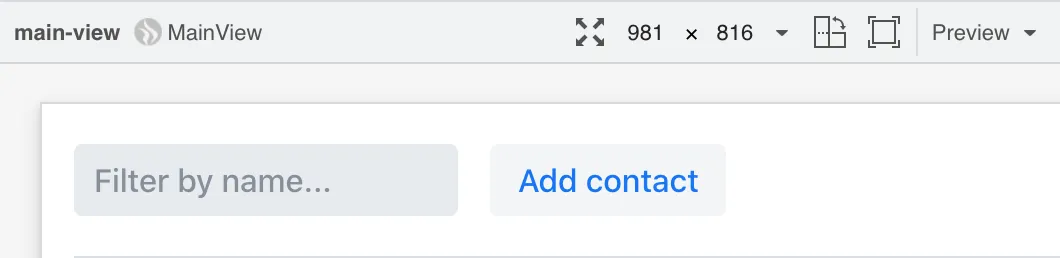
main-view
: MainView
.You can navigate to the companion file by clicking on its name in the toolbar. The file opens in your IDE.
If you don’t have a companion file for your design yet, you can create one manually. Here is a code snippet for a companion file that’s a valid starting point for any design. It has been written as if it was a companion to an imaginary my-design.js
. You have to adapt it by providing the correct values for your design to the Tag
and JsModule
annotations. The class names aren’t relevant for Designer.
import com.vaadin.flow.templatemodel.TemplateModel;
import com.vaadin.flow.component.Tag;
import com.vaadin.flow.component.dependency.JsModule;
import com.vaadin.flow.component.polymertemplate.PolymerTemplate;
@Tag("my-design")
@JsModule("./src/views/my-design.js")
public class MyDesign extends LitTemplate {
public MyDesign() {
// You can initialize any data required for the connected UI components here.
}
}
In general, any Java class is picked up by Designer as a companion file for the design, as long as the class meets the following requirements:
-
It’s a descendant of
com.vaadin.flow.component.Component
; -
It’s annotated with
com.vaadin.flow.component.Tag
annotation. The annotation’s value matches the design’s tag in custom element definition (for example,customElements.define('my-design', MyDesign)
); and -
The value of the
com.vaadin.flow.component.dependency.JsModule
annotation matches the design path.
If you have a specific need, you can customize the companion class to match your demands. You can learn more about connecting designs and Java classes in Flow documentation.
Connecting Components
Designer helps to connect the components used in the design to Java but before that can happen you need three things:
-
You need a companion file for the design. See the The Java Companion File for how to get one.
-
The component you want to connect to Java should have its
id
property set to a unique value (among all theid
property values in the same design). If itsid
is empty, Designer generates one for you. -
The project must have Vaadin Flow component integrations as dependencies. Those are needed to correctly set the type of the new field.
When a companion file for the design exists, you can connect components to Java using the Outline view. When you hover over a component in the Outline and the component has a Java API, a connection button appears on the same row with the component name. By clicking the connection button, you can connect the component to Java. This is shown in the following picture.
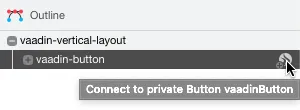
When the vaadin-button
in the previous picture is connected, the following field is added to the companion class:
@Id("vaadinButton")
private Button vaadinButton;
If the component has alternative Java APIs, you can right-click the connection button and choose an API from the context menu. For example, you might have your own Java class MyButton
that extends Vaadin’s Button
to provide some extra functionality. MyButton
is available in the context menu.
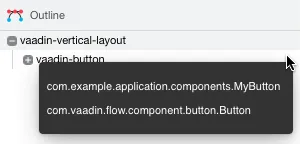
Note
| When you make changes in your Java companion file, it takes a few seconds to update the status of the connection buttons in the outline and the connection indicator on the toolbar. |
Flow uses the @Id
annotation to connect the UI component to the field. The value in the annotation must match the id
property of the component in the design. Otherwise, you are free to change the type, name and visibility of the field. Be careful not to break it for Flow.
Take a look at the Flow documentation to learn more about binding components in Flow.
You can disconnect a component by clicking the connection button of a connected component. Disconnecting a component erases the corresponding field from the companion class along with its @Id
annotation.
You shouldn’t have more than one companion class for a design, or more than one field annotated with the same @Id
value, but if you do, all of them are shown in the Java checkbox tooltip so that you can locate them to fix the problem manually.
Connecting Data
You can also bind data from Java to the UI. Designer provides you with a starting point by adding the template model inner class into the companion file when the file is created. You can learn more about binding data to designs in Flow documentation.
3FF26525-DCDC-4B25-A8E4-7C5E9DD98916