Protect Services
Flow views are already well protected, but you should consider protecting the services as well. This is important if you have views that allow users with different roles to do different things. If you, for instance, forget to disable a button for users lacking a particular role, and don’t protect your services, you have created a privilege escalation.
In this guide, you’ll learn how to control access to services by using Spring method security. A hands-on mini-tutorial at the end will help you apply these concepts in a real Vaadin application.
Introducing Method Security
In Vaadin Flow, services are standard Spring beans that are injected into views and used directly. Since services are accessed directly from views, they must be secured at the method level using Spring method security.
Spring Security protects services by creating a proxy that intercepts method calls. This ensures access control is enforced before execution, as shown in the following diagram:
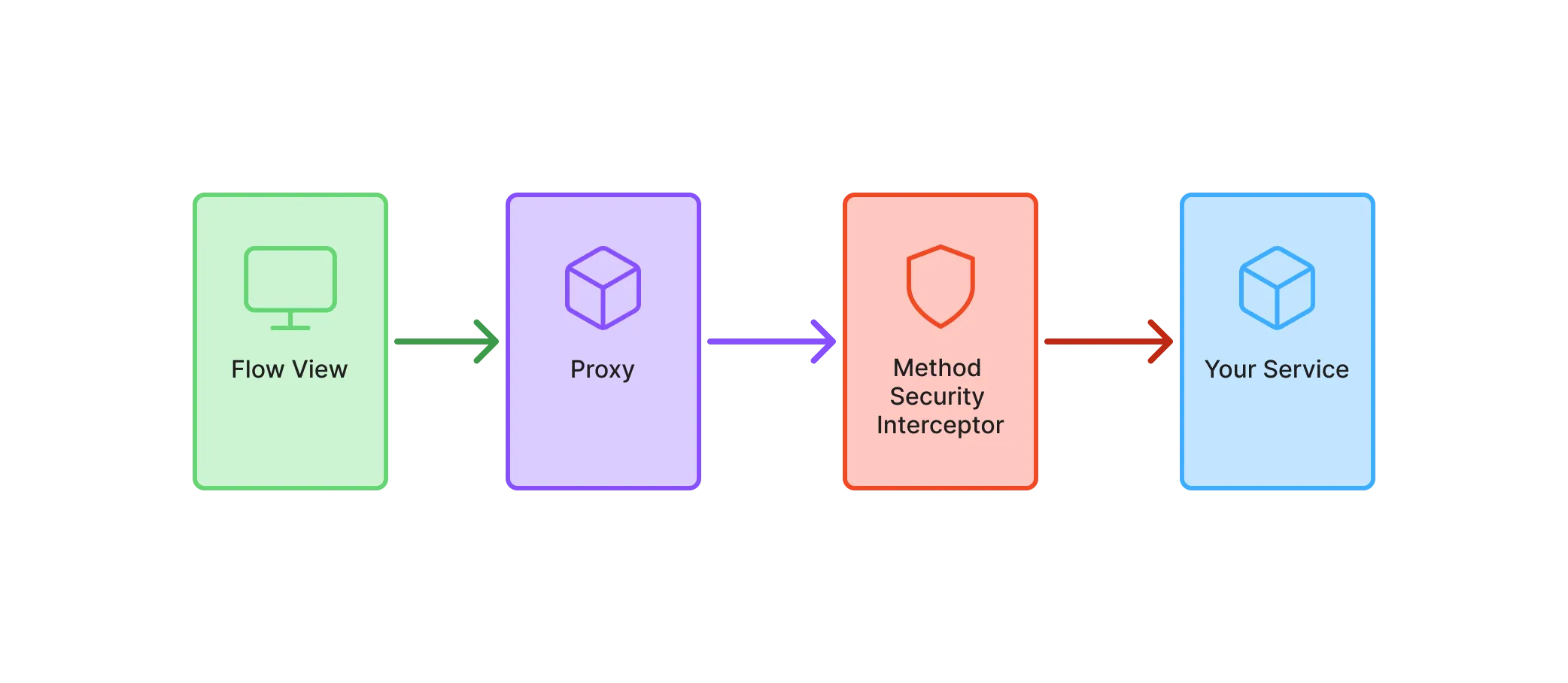
In this guide, you’ll only learn the minimum to get started with Spring method security in a Vaadin application. For more in-depth information, see the Spring Security Reference Manual.
Enabling Method Security
To enable method security, add @EnableMethodSecurity
to your security configuration class:
@EnableWebSecurity
@EnableMethodSecurity
@Configuration
class SecurityConfig extends VaadinWebSecurity {
@Override
protected void configure(HttpSecurity http) throws Exception {
super.configure(http);
setLoginView(http, LoginView.class);
}
...
}
Caution
|
Test the method security
Without @EnableMethodSecurity , all services remain unprotected — even if you annotate methods with security rules! Always verify that method security is enabled with automatic tests. A guide showing you how to do this in a Vaadin application is planned, but not yet written. In the meantime, refer to the Spring Reference Manual.
|
Securing the Services
Spring Security uses different annotations to secure your services. The most flexible ones, which are enabled by default, are @PreAuthorize
, @PostAuthorize
, @PreFilter
, and @PostFilter
. In this guide, you’ll only learn how to use @PreAuthorize
.
You can annotate both service classes and individual service methods. An annotation placed on the class applies to all public methods of the class. An annotation placed on a method overrides any annotation on the class.
@PreAuthorize
takes as its single argument a Spring Expression Language (SpEL) expression that must evaluate to true
to grant access. Although you can do some quite advanced things with SpEL, the most common methods you’ll want to use are:
-
permitAll
allows anyone to call the method. -
isAuthenticated
allows any authenticated user to call the method. -
hasRole
/hasAnyRole
allows users having the roles specified to call the method. -
denyAll
prevents anyone from calling the method.
You use the SpEL methods like this:
@Service
@PreAuthorize("isAuthenticated()") 1
public class ProtectedService {
public MyData callableByAllUsers() { 2
}
@PreAuthorize("hasRole('" + Roles.ADMIN + "')") 3
public void callableByAdminsOnly(MyData data) {
}
}
-
Allows all authenticated users to call the service by default.
-
Inherits its access permissions from the class-level annotation.
-
Overrides the class-level annotation to allow access to administrators only. Note the single quotes
'
around the role name.
Try It
In this mini-tutorial, you’ll learn how to use Spring method security in a real Vaadin application. The tutorial uses the project from the Protect Views guide. If you haven’t completed that tutorial yet, do it now before proceeding.
Enable Method Security
Add @EnableMethodSecurity
to SecurityConfig
:
@EnableWebSecurity
@EnableMethodSecurity
@Configuration
class SecurityConfig extends VaadinWebSecurity {
...
}
Secure the Todo Service
In an earlier tutorial, you made the task list read-only for users, allowing only admins to create tasks.
Open TodoService
and add @PreAuthorize
annotations like this:
@Service
@PreAuthorize("isAuthenticated()")
@Transactional(propagation = Propagation.REQUIRES_NEW)
public class TodoService {
...
@PreAuthorize("hasRole('" + Roles.ADMIN + "')")
public void createTodo(String description, @Nullable LocalDate dueDate) {
// ...
}
public List<Todo> list(Pageable pageable) {
// ...
}
}
Restart the application and open your browser at: http://localhost:8080
Log in as ADMIN
and create some tasks. Everything should work as before.
Break the Task List
To see that the service is actually protected, you’re going to break the task list. Open TodoView
and comment out the lines that check whether the user is an admin or not:
@Route("")
@PageTitle("Task List")
@Menu(order = 0, icon = "vaadin:clipboard-check", title = "Task List")
@PermitAll
public class TodoView extends Main {
public TodoView(TodoService todoService, Clock clock,
AuthenticationContext authenticationContext) {
// The rest of the constructor omitted
//if (authenticationContext.hasRole(Roles.ADMIN)) {
add(new ViewToolbar("Task List",
ViewToolbar.group(description, dueDate, createBtn)));
//} else {
// add(new ViewToolbar("Task List"));
//}
add(todoGrid);
}
...
}
Then go back to the browser, logout, and login as USER
. If you now try to create a task, you should get an error message.
Now change TodoView()
back again by removing the comments.
Final Thoughts
Your Vaadin application now has both secure views and secure services. However, it still uses in-memory authentication. You should replace it with a stronger storage mechanism.
Note
| A guide showing you how to do this in a Vaadin application is planned, but not yet written. In the meantime, refer to the Spring Security Reference Manual. |