Starting a Project
- Requirements
- Creating a Vaadin Project
- The Build File
- Compiling
- Running the Application
- Developing in the Eclipse IDE
- Going to Production
- Using Gradle Plugin Snapshot Versions
- Plugin Configuration Options
- Known Issues
Gradle is a popular build tool for Java, Groovy, Kotlin, and other projects. It’s an alternative to using Maven, and in many ways much simpler to use. It’s also more powerful, if that’s required. You can use it to build a Vaadin application, run it, and manage dependencies during development.
This tutorial describes how to create, compile, and run a Vaadin application using the Vaadin Gradle plugin. To run the application, the Gretty plugin is used to run it in an embedded web server.
For information about the general usage of Gradle, see the Gradle User Manual.
Requirements
To use the Vaadin Gradle plugin, your environment needs to meet the following requirements:
-
Windows, Mac, or Linux
-
Java SDK 11 or later
-
Gradle 5 or 6 (optional, because of the provided wrapper in the starter projects)
-
If you prefer to install it directly, you can find the instructions at https://gradle.org/install
-
-
Node.js and
npm
(optional, because they can also be installed locally to the project using the Vaadin Gradle plugin)
Note
|
Installing Gradle is optional
If you plan to use Vaadin’s Gradle-based starter projects, there is no need to install Gradle on your machine.
A Gradle Wrapper script is included in those starter projects.
This script manages the download and execution of Gradle locally for your project.
For more information on using Gradle Wrapper, see the Official Gradle Documentation.
|
Creating a Vaadin Project
The easiest way to create a new project is to clone a starter repository containing an application skeleton.
You can also take any existing Vaadin project and make a build.gradle
file for it, as described in "The Build File".
Cloning a Starter Repository
The following starter repositories are currently available:
Note
|
Clone the
The following repositories default to the V14 branch when you clone them.
If you plan to use them with the latest version of Vaadin, there two options.
You can either clone the master branch, or change to the master branch after you have cloned either of the starter projects.
master branch of the starter projects |
https://github.com/vaadin/base-starter-gradle
-
A simple web application project to be deployed as a WAR package. This example can also be used for Java EE, by changing the servlet dependency to
javax:javaee-api
and perhaps also adding the dependencycom.vaadin:vaadin-cdi
for CDI integration.git clone https://github.com/vaadin/base-starter-gradle my-project
https://github.com/vaadin/base-starter-spring-gradle
-
A web application project skeleton that uses Spring Boot.
git clone https://github.com/vaadin/base-starter-spring-gradle my-project
Starter Project Contents
When it has been cloned, the project should look as follows (imported into the Eclipse IDE):
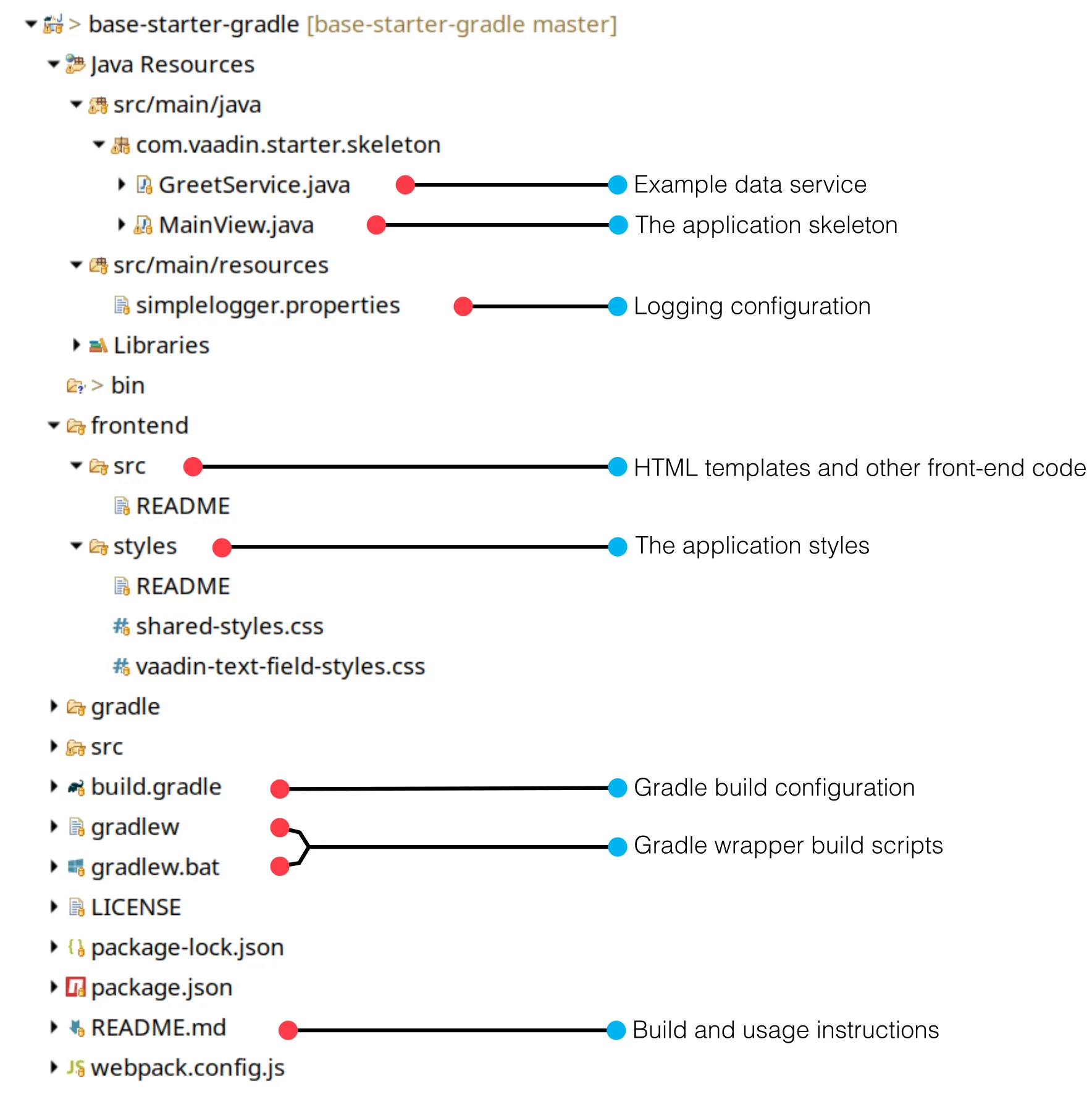
Note
|
Only the content of a simple web application starter is depicted
Only the contents of base-starter-gradle (a simple web application starter without Spring Boot) are presented in the preceding figure.
However, the Spring Boot-based starter project is little different, except that it has an additional class annotated with @SpringBootApplication .
If this is the first time you have and tried Vaadin with Spring Boot, see Using Vaadin with Spring Boot for more information.
|
The most important files and folders are as follows:
src/main/java/<package>/MainView.java
-
The application view class for the root route, built from components.
src/main/java/<package>/GreetService.java
-
A trivial service object to separate business data and logic from the view.
frontend/src
-
Folder for HTML templates and JavaScript code. See the
README
inside for more details. frontend/styles/shared-styles.css
-
Application-specific style sheets to style the look of the application.
frontend/styles/vaadin-text-field-styles.css
-
An example to modify the style of the
TextField
component. build.gradle
-
The Gradle build file as described later in The Build File.
gradlew
andgradlew.bat
-
Gradle Wrapper build scripts for Linux/Mac (
gradlew
) and Windows (gradlew.bat
). The build scripts enable the project to be built without having Gradle preinstalled. As the recommended way to execute any Gradle build is with the help of the Gradle Wrapper, we have also usedgradlew
instead ofgradle
throughout the documentation. However, thegradlew
andgradle
commands can be used interchangeably if you already have Gradle installed and you prefer to use your installed Gradle. You can find out more about the benefits of using Gradle Wrapper in the Official Gradle Documentation.
The Build File
As a minimum, the build.gradle
file needs to enable the Vaadin Gradle Plugin:
plugins {
id 'com.vaadin' version '20.0.0' (1)
// Optional
id 'org.gretty' version '3.0.3' (2)
id 'war' (3)
id 'groovy' (4)
}
-
Use the plugin version that matches the Vaadin version.
See the releases at github.com/vaadin/platform for the latest release.
To try the pre-release version of the Vaadin Gradle plugin, see Using plugin pre-release version
-
Use the Gretty embedded web server to run the application during development. See Running the Application for details.
-
Build a WAR package to deploy to a traditional servlet container. You also need to define the Servlet API using
providedCompile "javax.servlet:javax.servlet-api:3.1.0"
in the dependencies section. -
By default, the plugin supports Java. You can include Groovy or Kotlin as an optional plugin.
Vaadin Plugin Configuration
Vaadin Gradle plugin options are configured in a vaadin
block.
For development, the block is usually like this:
vaadin {
optimizeBundle = false
}
If the parameter is true
, the front-end bundle is optimized for all supported browsers, but compilation is much slower.
For configuration options, see plugin configuration options
Configuring Repositories
The repositories
section defines the locations to search for packages.
The repository that contains the Vaadin libraries is required as a minimum:
repositories {
mavenCentral()
maven { url = "https://maven.vaadin.com/vaadin-addons" }
}
If you want to try the Vaadin platform pre-release versions,you can add the following repository, as well:
repositories {
maven { url = "https://maven.vaadin.com/vaadin-prereleases" }
}
Note
|
Vaadin recommends using final releases
To avoid any inconsistencies, don’t use any pre-release versions in your production environment, especially snapshots.
Vaadin recommends using the latest major version.
Visit the https://vaadin.com/releases page for the latest releases.
|
You can use any Gradle repository definitions in the block. See Declaring repositories in the Gradle documentation for more information.
Configuring Dependencies
You need to add the vaadin-core
or vaadin
library as a Java dependency:
dependencies {
implementation "com.vaadin:vaadin-core:20.+"
}
When you specify a version of 20.+
, you are choosing to use the latest version of Vaadin, but you can also specify the exact version.
See Declaring dependencies in the Gradle documentation for further details.
Compiling
If you have defined the default tasks as described earlier, in Other Configuration, you can run:
./gradlew
on Windows:
gradlew
Note
|
The Unix style of running
To avoid unnecessary verbosity, only the Unix style of running gradlew is used for the rest of this document./gradlew is used for the rest of this document.
You need to replace it with gradlew if you are on a Windows machine.
|
Otherwise, the project builds with the standard build
task.
However, on the first build and also at other times when necessary, you need to build the Vaadin frontend.
./gradlew vaadinBuildFrontend build
Vaadin Tasks
The Vaadin-related tasks handled by the plugin are as follows:
vaadinPrepareFrontend
-
Checks that Node.js and
npm
are installed, copies front-end resources, and creates or updates thepackage.json
and Vite configuration files (vite.config.ts
andvite.generated.ts
). The front-end resources are inside.jar
dependencies, and copied tonode_modules
. vaadinBuildFrontend
-
Builds the front-end bundle with the
Vite
utility. Vaadin front-end resources, such as HTML, JavaScript, CSS, and images, are bundled to optimize loading the front-end. This task isn’t executed automatically on thebuild
and other targets, so you need to run it explicitly. vaadinClean
-
Cleans the project and removes
node_modules
,package-lock.json
,vite.generated.ts
,tsconfig.json
,types.d.ts
,pnpm-lock.yaml
andpnpmfile.js
. You need to run this task if you upgrade the Vaadin version, and in other similar situations.
To get the complete list of tasks handled by the configured plugins, enter:
./gradlew tasks
Running the Application
You use a Spring Boot-based starter (Vaadin with Spring Boot) to run the application during development in a similar way to any normal Spring Boot application.
This means you can run it either from the class containing the main()
method (normally annotated with @SpringBootApplication
), or by using Spring Boot’s Gradle plugin bootRun
task:
./gradlew bootRun
If you are using a simple web application (Vaadin without Spring Boot) to run the application during development, the Gradle plugin supports the Gretty plugin, which runs the application in an embedded web server. You can do this either in an IDE or at the command line.
One way to enable the Gretty plugin is in the plugin
section of the gradle.build
file, as in the starter project:
plugins {
...
id 'org.gretty' version '3.0.3'
}
You can configure Gretty further in an optional gretty
block:
gretty {
contextPath = "/" (1)
servletContainer = "jetty9.4" (2)
}
-
Sets the context path to the root path. The default context path contains the project name, so the URL would be
http://localhost:8080/myproject
(or whatever your project name is). -
Use Jetty as the servlet container, with the specified version.
The application is started with the appRun
task:
./gradlew appRun
The task compiles the application and starts the web server in http://localhost:8080/
(if the root context path is configured as described earlier).
You might need to add jcenter()
to the list of repositories to be able to run Gretty tasks, depending on the situation at the time you are following this documentation.
Some artifacts from jcenter()
haven’t yet been moved to mavenCentral()
.
In the future, this step becomes unnecessary:
repositories {
// should be removed in the future as jcenter() is obsolete.
jcenter()
}
See the Gretty documentation for a complete reference on using Gretty.
For issues when running the application in development mode, see Known Issues for possible solutions.
Developing in the Eclipse IDE
Gradle has first-class support in the Eclipse IDE, IDEA, NetBeans, and Android Studio, among others. The following section describes creating, importing, and developing a Vaadin Gradle project in the Eclipse IDE.
Importing a New Project
You create a new Vaadin project by cloning the repository on the command line and importing it to Eclipse as a Gradle project.
-
Clone the starter repository of your choice, as described earlier.
-
Select
. -
Enter or select the Project root directory.
-
Click Finish.
The project should appear in the Project Explorer and look as shown in Cloned Starter Project.
You should now see the Gradle Tasks tab. You can browse the available tasks.
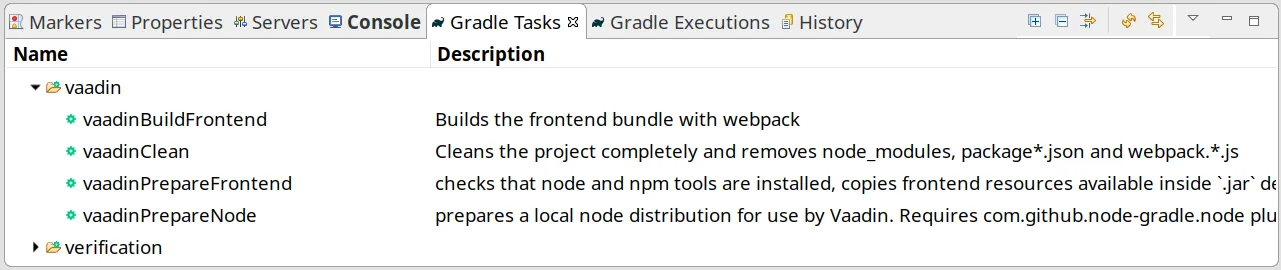
Running the Application
You can run the project using Gretty in an embedded web server.
-
Open the Gradle Tasks tab
-
Double-click the
gretty
→appRun
task-
The Gradle Executions tab opens and shows the build progress
-
-
When the
:apprun
task is running, openhttp://localhost:8080
in the browser. -
To stop the server, go to the Console tab and press any key.
Going to Production
To build a web application as a WAR package, you need the war
plugin.
You also need to enable it.
In build.gradle
, include the plugin and enable WAR build:
plugins {
...
id 'war'
}
war {
enabled = true
}
When doing a production-ready build, the Vaadin Gradle plugin transpiles the client-side dependencies to legacy browsers, as described in Deploying to Production.
You enable this by either setting it in build.gradle
or at the command line when invoking Gradle.
In build.gradle
:
vaadin {
productionMode = true
}
At the command line:
./gradlew -Pvaadin.productionMode=true war
Note
|
Spring Boot-specific configuration
If you are using Vaadin with Spring Boot, the default packaging for production would normally be the jar .
But, if you intend to package a Spring Boot application as a WAR to be deployed on a standalone container, such as tomcat , there are two additional steps you need to perform:
|
-
Your application class that’s annotated with
@SpringBootApplication
should extendSpringBootServletInitializer
and override theconfigure()
method:
@SpringBootApplication
public class DemoApplication extends SpringBootServletInitializer {
@Override
protected SpringApplicationBuilder configure(
SpringApplicationBuilder application) {
return application.sources(DemoApplication.class);
}
}
-
Adding the following dependency:
dependencies {
providedRuntime 'org.springframework.boot:spring-boot-starter-tomcat'
}
Using Gradle Plugin Snapshot Versions
A snapshot version of the plugin is pushed to the pre-release repository. This section is about trying the pre-release and snapshot versions of the Vaadin Gradle plugin itself, not Vaadin.
To use the pre-release plugin, add the vaadin-prereleases
repository to the project settings.gradle
file.
settings.gradle
is mostly used within multi-module projects, but it comes in handy for other configurations, too.
Thus, if you don’t already have it in your project, go ahead and create a plain text file called settings.gradle
next to your build.gradle
file (normally in the project root folder).
pluginManagement {
repositories {
maven { url = 'https://maven.vaadin.com/vaadin-prereleases' }
gradlePluginPortal()
}
}
The plugin then needs to be defined and applied in the build.gradle
file.
buildscript {
...
dependencies {
classpath group: 'com.vaadin',
name: 'vaadin-gradle-plugin',
version: '20.0-SNAPSHOT'
}
}
plugins {
...
}
apply plugin: 'com.vaadin'
Note
|
Remember to update the
Remove the part plugins blockid 'com.vaadin' version 'xyz' from the plugins block.
The plugin is applied by specifying apply plugin: 'com.vaadin' (as demonstrated in the preceding file extract).
|
Plugin Configuration Options
Here are all the configuration options with their default values:
productionMode: Boolean = false
-
Indicates that the application is running in production mode. Defaults to
false
. For production, the frontend is transpiled for older browsers and optimized, as described in Deploying to Production. Running thevaadinBuildFrontend
task automatically switches this totrue
, so there is no need to configure anything. frontendOutputDirectory: File = null
-
The folder where Vite should output
index.js
and other generated files. Defaults tonull
, which uses the automatically detected value of the main SourceSet, usuallybuild/resources/main/META-INF/VAADIN/webapp/
. npmFolder: File = project.projectDir
-
The folder where the
package.json
file is located. Defaults to the project root directory. generatedFolder: File(project.projectDir, "target/frontend")
-
The target folder for generated files used by Vite.
frontendDirectory: File(project.projectDir, "frontend")
-
The directory with the front-end source files of the project.
generateBundle: Boolean = true
-
Set to
true
to generate a bundle from the project front-end sources. runNpmInstall: Boolean = true
-
Run
npm install
after updating dependencies. generateEmbeddableWebComponents: Boolean = true
-
Generate web components from
WebComponentExporter
inheritors. frontendResourcesDirectory: File = File(project.projectDir, Constants.LOCAL_FRONTEND_RESOURCES_PATH)
-
Identifies the project front-end directory from where resources should be copied for use with Vite.
optimizeBundle: Boolean = true
-
Use byte code scanner strategy to discover front-end components.
pnpmEnable: Boolean = false
-
Use
pnpm
for installingnpm
front-end resources. Defaults tofalse
. useGlobalPnpm: Boolean = false
-
Use the globally installed
pnpm
tool or the default supportedpnpm
version. Defaults tofalse
. requireHomeNodeExec: Boolean = false
-
Force use of Vaadin home node executable. If it’s set to
true
, Vaadin home node is checked, and installed if absent. This is then be used instead of the globally or locally installed node. useDeprecatedV14Bootstrapping: Boolean = false
-
Run the application in legacy V14 bootstrap mode. Defaults to
false
. eagerServerLoad: Boolean = false
-
Add the initial User Interface Definition Language (UIDL) object to the bootstrap
index.html
. Defaults tofalse
. applicationProperties: File = File(project.projectDir, "src/main/resources/application.properties")
-
Application properties file in a Spring project.
openApiJsonFile: File = File(project.buildDir, "generated-resources/openapi.json")
-
Generated path of the OpenAPI JSON.
javaSourceFolder: File = File(project.projectDir, "src/main/java")
-
Java source folders for connect scanning.
generatedTsFolder: File = File(project.projectDir, "frontend/generated")
-
The folder where Flow puts TS API files for client projects.
nodeVersion: String = "v14.15.4"
-
The Node.js version to be used when Node.js is installed automatically by Vaadin, for example
"v14.15.4"
. Defaults to[FrontendTools.DEFAULT_NODE_VERSION]
. nodeDownloadRoot: String = "https://nodejs.org/dist/"
-
URL to download Node.js from. This can be needed in corporate environments where the Node.js download is provided from an intranet mirror. Defaults to
[NodeInstaller.DEFAULT_NODEJS_DOWNLOAD_ROOT]
. nodeAutoUpdate: Boolean = false
-
Flag to enable automatic update of the Node.js version installed in
~/.vaadin
if it’s older than the default or definednodeVersion
. resourceOutputDirectory: File = File(project.buildDir, "vaadin-generated")
-
The output directory for generated non-served resources, such as the token file. Defaults to
build/vaadin-generated
.
Known Issues
Spring Boot
When the list of dependencies causes the classpath to go over a set limit on Windows, the build automatically generates a JAR containing a manifest with the classpath.
Sometimes, when running a Spring Boot application, the resource loader doesn’t load the classpath packages correctly from the manifest.
The failed annotation scanning makes the required npm
packages unavailable.
You can fix this in two ways:
-
add the repository
mavenLocal()
to build file repositories -
specify the
vaadin.whitelisted-packages
property; see Vaadin Spring Configuration
FA18F1BF-2C67-4CCF-85A2-C3E4D7AECFDB