Font Icons
- Loading Icon Fonts
- Basic Use
- Using Font icons in HTML
- Using Font Icons in Other Text
- Custom Font Icons
Font icons are icons included in a font. Fonts have many advantages over bitmap images. Browsers are usually faster in rendering fonts than loading image files. Web fonts are vector graphics, so they are scalable. As font icons are text characters, you can define their color in CSS by the regular foreground color property.
Loading Icon Fonts
Vaadin currently comes with one custom icon font: Vaadin Icons. It is automatically included in the Valo theme.
If you use other icon fonts, as described in Custom Font Icons,
you need to load it with a font
mixin in Sass, as described in
"Loading Local Fonts".
Basic Use
Font icons are resources of type FontIcon, which implements the Resource interface. You can use these special resources for component icons and such, but not as embedded images, for example.
Each icon has a Unicode codepoint, by which you can use it. Vaadin Framework includes its own icon font, Vaadin Icons, which comes with an enumeration VaadinIcons with all the icons included in the font.
Most typically, you set a component icon as follows:
TextField name = new TextField("Name");
name.setIcon(VaadinIcons.USER);
layout.addComponent(name);
// Button allows specifying icon resource in constructor
Button ok = new Button("OK", VaadinIcons.CHECK);
layout.addComponent(ok);
The result is illustrated in Basic Use of Font Icons, with the color styling described next.
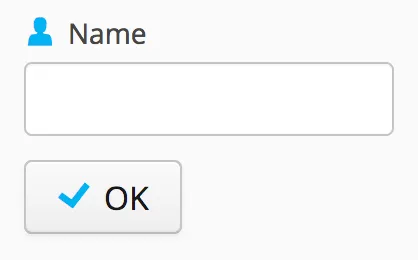
Styling the Icons
As font icons are regular text, you can specify their color with the color attribute in CSS to specify the foreground text color. All HTML elements that display icons in Vaadin have the v-icon style name.
.blueicon .v-icon {
color: blue;
}
If you use the font icon resources in other ways, such as in an Image component, the style name will be different.
Using Font icons in HTML
You can use font icons in HTML code, such as in a Label, by generating the HTML to display the icon with the getHtml() method.
Label label = new Label("I " +
VaadinIcons.HEART.getHtml() + Vaadin",
ContentMode.HTML);
label.addStyleName("redicon");
The result is illustrated in Using Font Icons in Label, with the color styling described next.
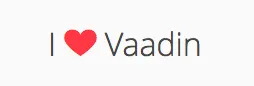
You could have set the font color in the label’s HTML code as well, or for all icons in the UI.
You can easily use font icons in HTML code in other ways as well. You just need to use the correct font family and then use the hex-formatted Unicode codepoint for the icon. See for example the implementation of the getHtml() method in FontAwesome:
@Override
public String getHtml() {
return "<span class=\"v-icon\" style=\"font-family: " +
getFontFamily() + ";\">&#x" +
Integer.toHexString(codepoint) + ";</span>";
}
Using Font Icons in Other Text
You can include a font icon in any text by its Unicode codepoint, which you can get with the getCodePoint() method. In such case, however, you need to use the same font for other text in the same string as well. The VaadinIcons provided with Valo theme includes a basic character set.
TextField amount = new TextField("Amount (in " +
new String(Character.toChars(
VaadinIcons.DOLLAR.getCodepoint())) +
")");
amount.addStyleName("amount");
layout.addComponent(amount);
You need to set the font family in CSS.
.v-caption-amount .v-captiontext {
font-family: Vaadin-Icons;
}
Custom Font Icons
You can easily use glyphs in existing fonts as icons, or create your own.
Creating New Icon Fonts With IcoMoon
You are free to use any of the many ways to create icons and embed them into fonts. Here, we give basic instructions for using the IcoMoon service, where you can pick icons from a large library of well-designed icons.
After you have selected the icons that you want in your font, you can download them in a ZIP package. The package contains the icons in multiple formats, including WOFF, TTF, EOT, and SVG. Not all browsers support any one of them, so all are needed to support all the common browsers. Extract the fonts folder from the package to under your theme.
See "Loading Local Fonts" for instructions for loading a custom font.
Implementing FontIcon
You can define a font icon for any font available in the browser by implementing the FontIcon interface. The normal pattern for implementing it is to implement an enumeration for all the symbols available in the font. See the implementation of VaadinIcons for more details.
You need a FontIcon API for the icons. In the following, we define a font icon using a normal sans-serif font built-in in the browser.
// Font icon definition with a single symbol
public enum MyFontIcon implements FontIcon {
EURO(0x20AC);
private int codepoint;
MyFontIcon(int codepoint) {
this.codepoint = codepoint;
}
@Override
public String getMIMEType() {
throw new UnsupportedOperationException(
FontIcon.class.getSimpleName()
+ " should not be used where a MIME type is needed.");
}
@Override
public String getFontFamily() {
return "sans-serif";
}
@Override
public int getCodepoint() {
return codepoint;
}
@Override
public String getHtml() {
return "<span class=\"v-icon\" style=\"font-family: " +
getFontFamily() + ";\">&#x" +
Integer.toHexString(codepoint) + ";</span>";
}
}
Then you can use it as usual:
TextField name = new TextField("Amount");
name.setIcon(MyFontIcon.EURO);
layout.addComponent(name);
You could make the implementation a class as well, instead of an enumeration, to allow other ways to specify the icons.