Interfaces and Abstractions
Vaadin user interface components are built on a skeleton of interfaces and abstract classes that define and implement the features common to all components and the basic logic how the component states are serialized between the server and the client.
This section gives details on the basic component interfaces and abstractions. The layout and other component container abstractions are described in "Managing Layout". The interfaces that define the Vaadin data model are described in "Binding Components to Data".
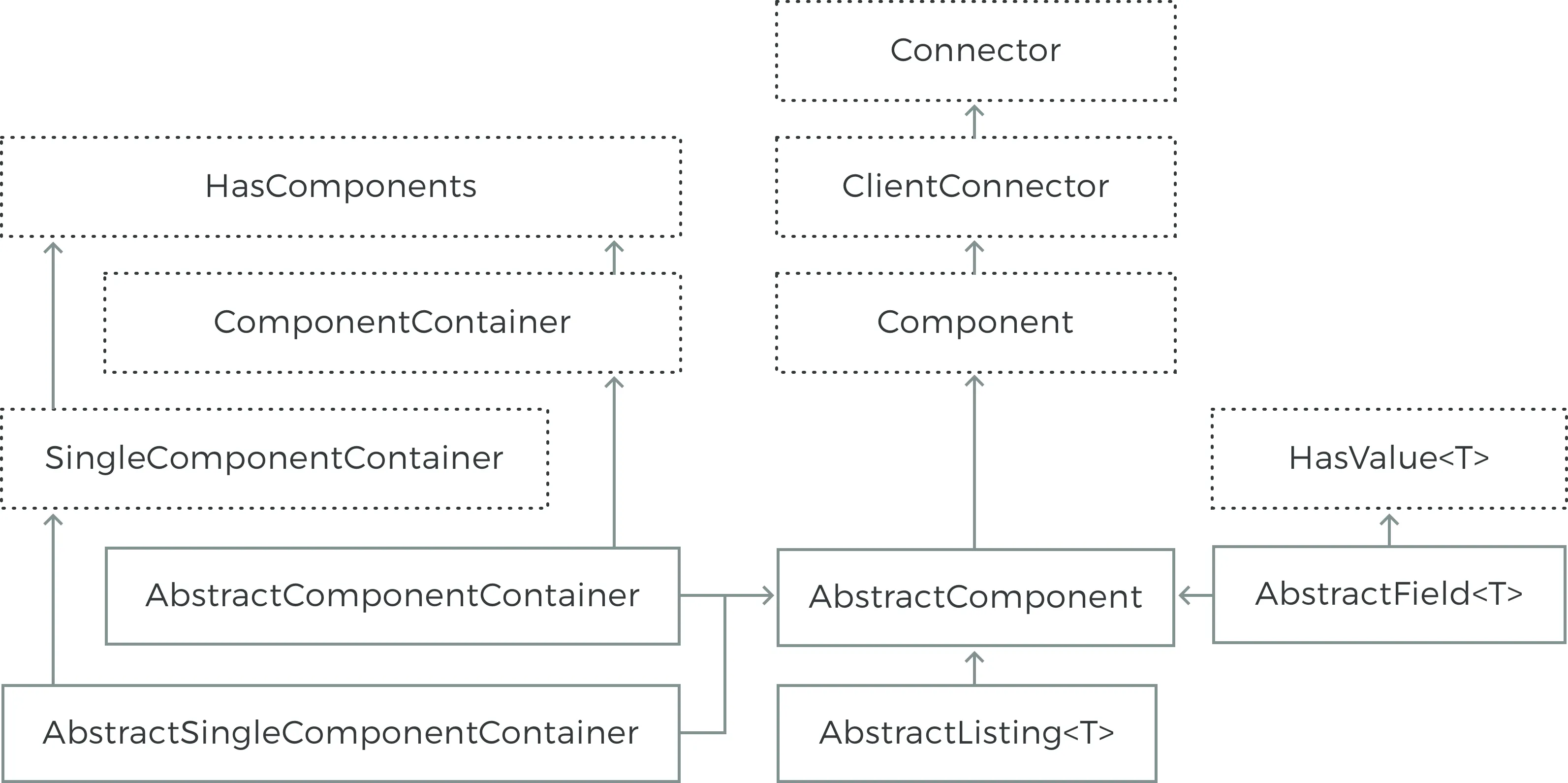
All components are connectors that connect to the client-side widgets.
In addition to the interfaces defined within the Vaadin framework, all components implement the java.io.Serializable interface to allow serialization. Serialization is needed in many clustering and cloud computing solutions.
Component Interface
The Component interface is paired with the AbstractComponent class, which implements all the methods defined in the interface.
Component Tree Management
Components are laid out in the user interface hierarchically. The layout is managed by layout components, or more generally components that implement the ComponentContainer interface. Such a container is the parent of the contained components.
The getParent() method allows retrieving the parent component of a component. While there is a setParent(), you rarely need it as you usually add components with the addComponent() method of the ComponentContainer interface, which automatically sets the parent.
A component does not know its parent when the component is still being created, so you can not refer to the parent in the constructor with getParent().
Attaching a component to an UI triggers a call to its attach() method. Correspondingly, removing a component from a container triggers calling the detach() method. If the parent of an added component is already connected to the UI, the attach() is called immediately from setParent().
public class AttachExample extends CustomComponent {
public AttachExample() {
}
@Override
public void attach() {
super.attach(); // Must call.
// Now we know who ultimately owns us.
ClassResource r = new ClassResource("smiley.jpg");
Image image = new Image("Image:", r);
setCompositionRoot(image);
}
}
The attachment logic is implemented in AbstractComponent, as described in AbstractComponent.