TextField
TextField is one of the most commonly used user interface components. It is a field that allows entering textual values with keyboard.
The following example creates a simple text field:
// Create a text field
TextField tf = new TextField("A Field");
// Put some initial content in it
tf.setValue("Stuff in the field");
The result is shown in TextField Example.
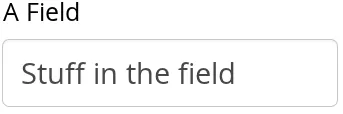
Value changes are handled by adding a listener using addValueChangeListener() method, as in most other fields. The value can be acquired with getValue() directly from the text field or from the parameter passed to the event listener.
// Handle changes in the value
tf.addValueChangeListener(event ->
// Do something with the value
Notification.show("Value is: " + event.getValue()));
TextField edits String values, but you can use Binder to bind it to any property type that has a proper converter, as described in "Conversion".
Much of the API of TextField is defined in AbstractTextField, which allows different kinds of text input fields, which do not share all the features of the single-line text fields.
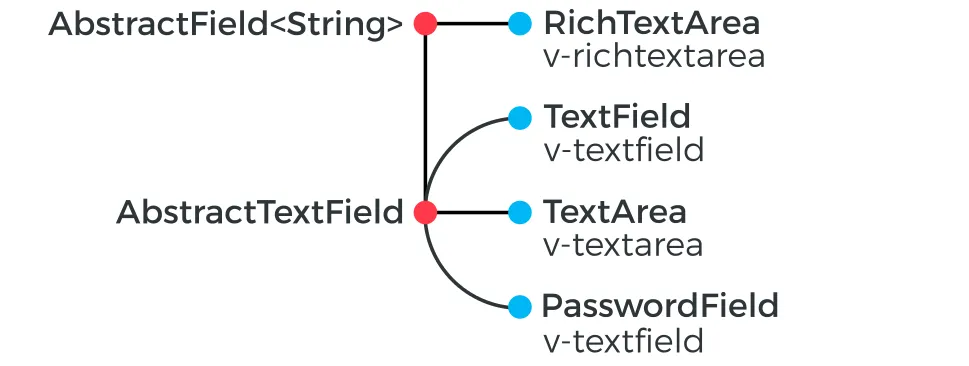
String Length
The setMaxLength() method sets the maximum length of the input string so that the browser prevents the user from entering a longer one. As a security feature, the input value is automatically truncated on the server-side, as the maximum length setting could be bypassed on the client-side. The maximum length property is defined at AbstractTextField level.
Notice that the maximum length setting does not affect the width of the field. You can set the width with setWidth(), as with other components. Using em widths is recommended to better approximate the proper width in relation to the size of the used font, but the em width is not exactly the width of a letter and varies by browser and operating system. There is no standard way in HTML for setting the width exactly to a number of letters (in a monospaced font).
Configuring the Granularity of Value Change Events
Often you want to control how frequently TextField value changes are transmitted to the server. Sometimes the changes should be sent only after the field loses focus. In the other extreme, it can sometimes be useful to receive events every time the user presses a key.
The value change event mode defines how quickly the changes are transmitted to the server and cause a server-side event. Lazier change events allow sending larger changes in one event if the user is typing fast, thereby reducing server requests.
You can set the text change event mode of a TextField with setValueChangeMode(). The allowed modes are defined in ValueChangeMode enum and are as follows:
- ValueChangeMode.LAZY(default)
-
An event is triggered when there is a pause in editing the text. The length of the pause can be modified with setValueChangeTimeout().
This is the default mode.
- ValueChangeMode.TIMEOUT
-
A text change in the user interface causes the event to be communicated to the application after a timeout period. If more changes are made during this period, the event sent to the server-side includes the changes made up to the last change. The length of the timeout can be set with setValueChangeTimeout().
- ValueChangeMode.EAGER
-
The ValueChangeEvent is triggered immediately for every change in the text content, typically caused by a key press. The requests are separate and are processed sequentially one after another. Change events are nevertheless communicated asynchronously to the server, so further input can be typed while event requests are being processed.
- ValueChangeMode.BLUR
-
The ValueChangeEvent is fired, after the field has lost focus.
// Text field with maximum length
TextField tf = new TextField("My Eventful Field");
tf.setValue("Initial content");
tf.setMaxLength(20);
// Counter for input length
Label counter = new Label();
counter.setValue(tf.getValue().length() +
" of " + tf.getMaxLength());
// Display the current length interactively in the counter
tf.addValueChangeListener(event -> {
int len = event.getValue().length();
counter.setValue(len + " of " + tf.getMaxLength());
});
tf.setValueChangeMode(ValueChangeMode.EAGER);
The result is shown in Text Change Events.
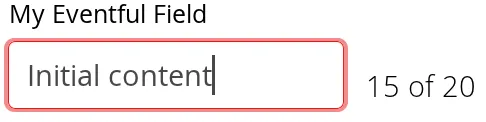
CSS Style Rules
.v-textfield { }
The HTML structure of TextField is extremely simple, consisting only of an element with the v-textfield style.
For example, the following custom style uses dashed border:
.v-textfield-dashing {
border: thin dashed;
}
The result is shown in Styling TextField with CSS.
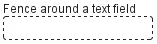
The style name for TextField is also used in several components that contain a text input field, even if the text input is not an actual TextField. This ensures that the style of different text input boxes is similar.