Overview
A Vaadin Framework application runs as a Java Servlet in a servlet container. The Java Servlet API is, however, hidden behind the framework. The user interface of the application is implemented as a UI class, which needs to create and manage the user interface components that make up the user interface. User input is handled with event listeners, although it is also possible to bind the user interface components directly to data. The visual style of the application is defined in themes as CSS or Sass. Icons, other images, and downloadable files are handled as resources, which can be external or served by the application server or the application itself.
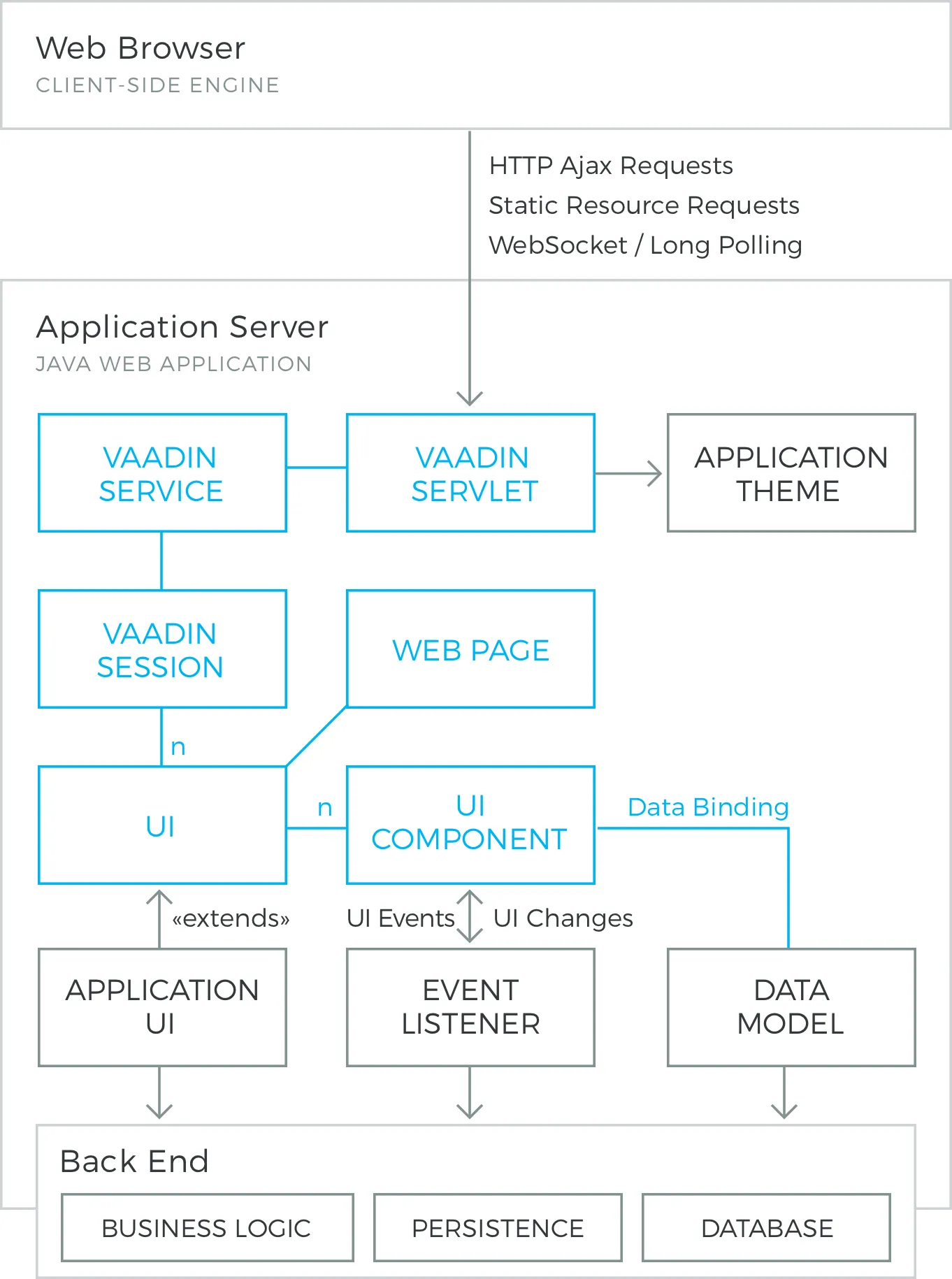
Vaadin Framework Application Architecture illustrates the basic architecture of an application made with the Vaadin Framework, with all the major elements, which are introduced below and discussed in detail in this chapter.
First of all, a Vaadin Framework application must have one or more UI classes that extend the abstract com.vaadin.ui.UI class and implement the init() method. A custom theme can be defined as an annotation for the UI.
@Theme("hellotheme")
public class HelloWorld extends UI {
protected void init(VaadinRequest request) {
... initialization code goes here ...
}
}
A UI is a viewport to the application running in a web page. A web page can actually have multiple such UIs within it. Such situation is typical especially with portlets in a portal. An application can run in multiple browser windows, each having a distinct UI instance. The UIs of an application can be the same UI class or different.
Vaadin Framework handles servlet requests internally and associates the requests with user sessions and a UI state. Because of this, you can develop applications with Vaadin Framework much like you would develop desktop applications.
The most important task in the initialization is the creation of the initial user interface. This, and the deployment of a UI as a Java Servlet in the Servlet container, as described in "Deploying an Application", are the minimal requirements for an application.
Below is a short overview of the other basic elements of an application besides UI:
- UI
-
A UI represents an HTML fragment in which a Vaadin application runs in a web page. It typically fills the entire page, but can also be just a part of a page. You normally develop an application with Vaadin Framework by extending the UI class and adding content to it. A UI is essentially a viewport connected to a user session of an application, and you can have many such views, especially in a multi-window application. Normally, when the user opens a new page with the URL of the UI, a new UI (and the associated Page object) is automatically created for it. All of them share the same user session.
The current UI object can be accessed globally with UI.getCurrent(). The static method returns the thread-local UI instance for the currently processed request (see "ThreadLocal Pattern") .
- Page
-
A UI is associated with a Page object that represents the web page as well as the browser window in which the UI runs.
The Page object for the currently processed request can be accessed globally from a Vaadin application with Page.getCurrent(). This is equivalent to calling UI.getCurrent().getPage().
- Vaadin Session
-
A VaadinSession object represents a user session with one or more UIs open in the application. A session starts when a user first opens a UI of a Vaadin application, and closes when the session expires in the server or when it is closed explicitly.
- User Interface Components
-
The user interface consists of components that are created by the application. They are laid out hierarchically using special layout components, with a content root layout at the top of the hierarchy. User interaction with the components causes events related to the component, which the application can handle. Field components are intended for inputting values and can be directly bound to data using the data model of the framework. You can make your own user interface components through either inheritance or composition. For a thorough reference of user interface components, see "User Interface Components", for layout components, see "Managing Layout", and for compositing components, see "Composition with Composite and CustomComponent".
- Events and Listeners
-
Vaadin Framework follows an event-driven programming paradigm, in which events, and listeners that handle the events, are the basis of handling user interaction in an application (although also server push is possible as described in "Server Push"). "Events and Listeners" gave an introduction to events and listeners from an architectural point-of-view, while "Handling Events with Listeners" later in this chapter takes a more practical view.
- Resources
-
A user interface can display images or have links to web pages or downloadable documents. These are handled as resources, which can be external or provided by the web server or the application itself. "Images and Other Resources" gives a practical overview of the different types of resources.
- Themes
-
The presentation and logic of the user interface are separated. While the UI logic is handled as Java code, the presentation is defined in themes as CSS or SCSS. Vaadin includes some built-in themes. User-defined themes can, in addition to style sheets, include HTML templates that define custom layouts and other theme resources, such as images. Themes are discussed in detail in "Themes", custom layouts in "Custom Layouts", and theme resources in "Theme Resources".
- Data Binding
-
With data binding, any field component in Vaadin Framework can be bound to the properties of business objects such as JavaBeans and grouped together as forms. The components can get their values from and update user input to the data model directly, without the need for any control code. Similarly, any select component can be bound to a data provider, fetching its items from a Java Collection or a backend such as an SQL database. For a complete overview of data binding in Vaadin, please refer to "Binding Components to Data".