TreeGrid
Overview
TreeGrid is for displaying hierarchical tabular data laid out in rows and columns. It is otherwise identical to the Grid component, but it adds the possibility to show hierarchical data, allowing the user to expand and collapse nodes to show or hide data.
See the documentation for "Grid" for all the shared features between Grid and TreeGrid.
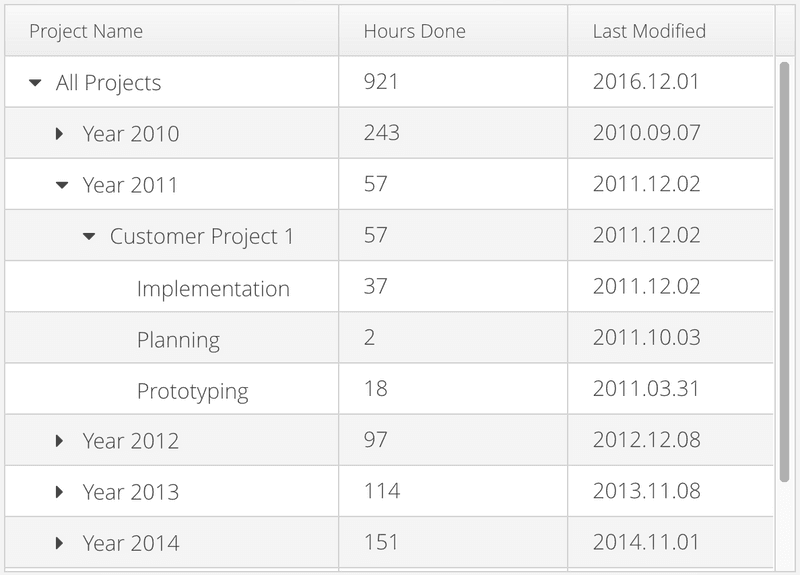
Binding to Data
TreeGrid is used by binding it to a hierarchical data provider. The data provider can be based on in-memory or back end data. For in-memory data, the TreeDataProvider can be used, and for loading data from a back end, you need to implement three methods from the HierarchicalDataProvider interface. Usage of both data providers is described in "Hierarchical Data".
Populating a TreeGrid with in-memory data can be done as follows
// Initialize a TreeGrid and set in-memory data
TreeGrid<Project> treeGrid = new TreeGrid<>();
treeGrid.setItems(getRootProjects(), Project::getSubProjects);
// The first column gets the hierarchy indicator by default
treeGrid.addColumn(Project::getName).setCaption("Project Name");
treeGrid.addColumn(Project::getHoursDone).setCaption("Hours Done");
treeGrid.addColumn(Project::getLastModified).setCaption("Last Modified");
The TreeData class can be used to build the hierarchical data structure, and it can then be passed on to TreeDataProvider. It is simply a hierarchical collection, that the data provider uses to populate the TreeGrid.
The setItems method in TreeGrid can be used to set the root level items. Internally an TreeDataProvider with TreeData is used. If at any time you want to modify the in-memory data in the grid, you may do it as follows
TreeDataProvider<Project> dataProvider = (TreeDataProvider<Project>) treeGrid.getDataProvider();
TreeData<Project> data = dataProvider.getTreeData();
// add new items
data.addItem(null, newProject);
data.addItems(newProject, newProject.getChildren());
// after adding / removing data, data provider needs to be refreshed
dataProvider.refreshAll();
Note that for adding or removing nodes, you always need to call the refreshAll method in the data provider you are using. The refreshItem method can only be used when just the data for that item is updated, but not for updates that add or remove items.
Expanding and Collapsing Nodes
TreeGrid nodes that have children can be expanded and collapsed by either user interaction or through the server-side API:
// Expands a child project. If the child project is not yet
// in the visible hierarchy, nothing will be shown.
treeGrid.expand(childProject);
// Expands the root project. If child project now becomes
// visible it is also expanded into view.
treeGrid.expand(rootProject);
// Collapses the child project.
treeGrid.collapse(childProject);
To use the server-side API with a backend data provider the hashCode and equals methods for the node’s type must be implemented so that when the desired node is retrieved from the backend it can be correctly matched with the object passed to either expand or collapse.
Changing the Hierarchy Column
By default, the TreeGrid shows the hierarchy indicator by default in the first column of the grid. You can change it with with the setHierarchyColumn, method, that takes as a parameter the column’s ID specified with the setId method in Column.
// the first column gets the hierarchy indicator by default
treeGrid.addColumn(Project::getLastModified).setCaption("Last Modified");
treeGrid.addColumn(Project::getName).setCaption("Project Name").setId("name");
treeGrid.addColumn(Project::getHoursDone).setCaption("Hours Done");
treeGrid.setHierarchyColumn("name");
Prevent Node Collapsing
TreeGrid supports setting a callback method that can allow or prevent the user from collapsing an expanded node. It can be set with setItemCollapseAllowedProvider method, that takes a SerializablePredicate. For nodes that cannot be collapsed, the collapse-disabled class name is applied to the expansion element
Avoid doing any heavy operations in the method, since it is called for each item when it is being sent to the client.
Example using a predefined set of persons that can not be collapsed:
Set<Person> alwaysExpanded;
personTreeGrid.setItemCollapseAllowedProvider(person ->
!alwaysExpanded.contains(person));
Listening to Events
In addition to supporting all the listeners of the standard Grid, TreeGrid supports listening to the expansion and collapsing of items in its hierarchy. The expand and collapse listeners can be added as follows:
treeGrid.addExpandListener(event -> log("Item expanded: " + event.getExpandedItem()));
treeGrid.addCollapseListener(event -> log("Item collapsed: " + event.getCollapsedItem()));
The return types of the methods getExpandedItem
and getCollapsedItem
are the same as the type of the TreeGrid the events originated from.
Note that collapse listeners will not be triggered for any expanded subtrees of the collapsed item.
Keyboard Navigation and Focus Handling in TreeGrid
As opposed to Grid, individual cells are not focusable in TreeGrid, and only whole rows receive focus. The user can navigate through rows with Up and Down, collapse rows with Left, and expand them with Right.