Building the UI
- Application Architecture
- Compositing Components
- View Navigation
- Accessing UI, Page, Session, and Service
Vaadin Framework user interfaces are built hierarchically from components, so that the leaf components are contained within layout components and other component containers. Building the hierarchy starts from the top (or bottom - whichever way you like to think about it), from the UI class of the application. You normally set a layout component as the content of the UI and fill it with other components.
public class MyHierarchicalUI extends UI {
@Override
protected void init(VaadinRequest request) {
// The root of the component hierarchy
VerticalLayout content = new VerticalLayout();
content.setSizeFull(); // Use entire window
setContent(content); // Attach to the UI
// Add some component
content.addComponent(new Label("<b>Hello!</b> - How are you?",
ContentMode.HTML));
Grid<Person> grid = new Grid<>();
grid.setCaption("My Grid");
grid.setItems(GridExample.generateContent());
grid.setSizeFull();
content.addComponent(grid);
content.setExpandRatio(grid, 1); // Expand to fill
}
}
The component hierarchy is illustrated in Schematic diagram of the UI.
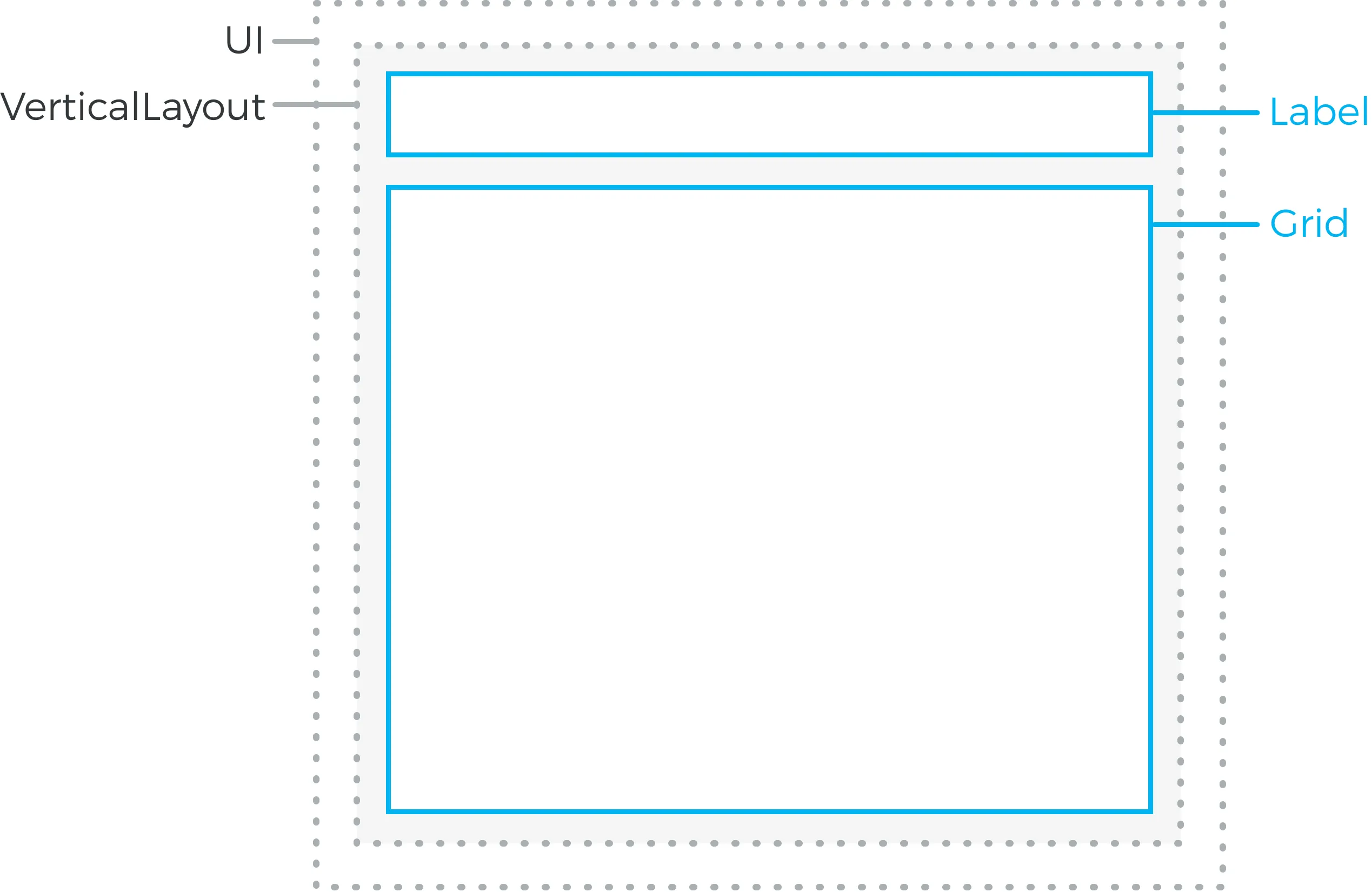
The actual UI is shown in Simple hierarchical UI.
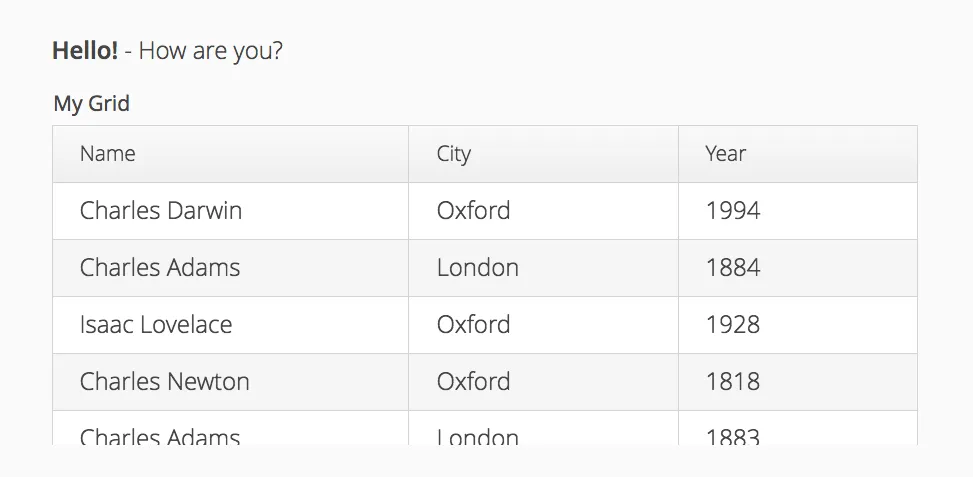
Instead of building the layout in Java, you can also use a declarative design, as described later in "Designing UIs Declaratively". The examples given for the declarative layouts give exactly the same UI layout as built from the components above. The easiest way to create declarative designs is to use Vaadin Designer.
The built-in components are described in "User Interface Components" and the layout components in "Managing Layout".
The example application described above just is, it does not do anything. User interaction is handled with event listeners, as described a bit later in "Handling Events with Listeners".
Application Architecture
Once your application grows beyond a dozen or so lines, which is usually quite soon, you need to start considering the application architecture more closely. You are free to use any object-oriented techniques available in Java to organize your code in methods, classes, packages, and libraries. An architecture defines how these modules communicate together and what sort of dependencies they have between them. It also defines the scope of the application. The scope of this book, however, only gives a possibility to mention some of the most common architectural patterns in Vaadin applications.
The subsequent sections describe some basic application patterns. For more information about common architectures, see "Advanced Application Architectures", which discusses layered architectures, the Model-View-Presenter (MVP) pattern, and so forth.
Compositing Components
User interfaces typically contain many user interface components in a layout hierarchy. Vaadin provides many layout components for laying contained components vertically, horizontally, in a grid, and in many other ways. You can extend layout components to create composite components.
class MyView extends VerticalLayout {
TextField entry = new TextField("Enter this");
Label display = new Label("See this");
Button click = new Button("Click This");
public MyView() {
addComponent(entry);
addComponent(display);
addComponent(click);
setSizeFull();
addStyleName("myview");
}
}
// Create an instance of MyView
Layout myview = new MyView();
While extending layouts is an easy way to make component composition, it is a good practice to encapsulate implementation details, such as the exact layout component used. Otherwise, the users of such a composite could begin to rely on such implementation details, which would make changes harder. For this purpose, Vaadin has the special wrappers Composite and CustomComponent, which hide the content representation.
class MyView extends CustomComponent {
TextField entry = new TextField("Enter this");
Label display = new Label("See this");
Button click = new Button("Click This");
public MyView() {
Layout layout = new VerticalLayout();
layout.addComponent(entry);
layout.addComponent(display);
layout.addComponent(click);
setCompositionRoot(layout);
setSizeFull();
}
}
// Create an instance of MyView
MyView myview = new MyView();
For a more detailed description of Composite and CustomComponent, see "Composition with Composite and CustomComponent".
View Navigation
While the simplest applications have just one view (or screen), most of them often require several. Even in a single view, you often want to have sub-views, for example to display different content. Navigation Between Views illustrates a typical navigation between different top-level views of an application, and a main view with sub-views.
The Navigator described in "Navigating in an Application" is a view manager that provides a flexible way to navigate between views and sub-views, while managing the URI fragment in the page URL to allow bookmarking, linking, and going back in the browser history.
Often Vaadin application views are part of something bigger. In such cases, you may need to integrate the Vaadin applications with the other website. You can use the embedding techniques described in "Embedding UIs in Web Pages".
Accessing UI, Page, Session, and Service
You can get the UI and the page to which a component is attached to with getUI() and getPage().
However, the values are null until the component is attached to the UI, and typically, when you need it in constructors, it is not. It is therefore preferable to access the current UI, page, session, and service objects from anywhere in the application using the static getCurrent() methods in the respective UI, Page, VaadinSession, and VaadinService classes.
// Set the default locale of the UI
UI.getCurrent().setLocale(new Locale("en"));
// Set the page title (window or tab caption)
Page.getCurrent().setTitle("My Page");
// Set a session attribute
VaadinSession.getCurrent().setAttribute("myattrib", "hello");
// Access the HTTP service parameters
File baseDir = VaadinService.getCurrent().getBaseDirectory();
You can get the page and the session also from a UI with getPage() and getSession() and the service from VaadinSession with getService().
The static methods use the built-in ThreadLocal support in the classes. The pattern is described in "ThreadLocal Pattern".