Vaadin Flow Quick Start
Caution
|
This tutorial is for Vaadin 14.
If this is your first time trying out Vaadin, you should read the same tutorial for the latest Vaadin version instead.
|
Vaadin Flow enables you to quickly build web apps in pure Java, without writing any HTML or JavaScript.
In this tutorial, you learn how to build a small but fully functional Todo application using Vaadin Flow.
What You Need
-
About 5 minutes
-
JDK 8 or higher (For example, Eclipse Temurin JDK).
Step 1: Download a Vaadin Project
Unpack the downloaded zip into a folder on your computer, and import the project in the IDE of your choice. (Alternatively, open the project in an online IDE).
Step 2: Add Your Code
Open src/main/java/org/vaadin/example/MainView.java
.
Replace the code in MainView with the code below:
package org.vaadin.example;
import com.vaadin.flow.component.Key;
import com.vaadin.flow.component.button.Button;
import com.vaadin.flow.component.checkbox.Checkbox;
import com.vaadin.flow.component.html.H1;
import com.vaadin.flow.component.orderedlayout.HorizontalLayout;
import com.vaadin.flow.component.orderedlayout.VerticalLayout;
import com.vaadin.flow.component.textfield.TextField;
import com.vaadin.flow.router.Route;
@Route("") 1
public class MainView extends VerticalLayout { 2
public MainView() {
VerticalLayout todosList = new VerticalLayout(); 3
TextField taskField = new TextField(); 4
Button addButton = new Button("Add"); 5
addButton.addClickListener(click -> { 6
Checkbox checkbox = new Checkbox(taskField.getValue());
todosList.add(checkbox);
});
addButton.addClickShortcut(Key.ENTER); 7
add( 8
new H1("Vaadin Todo"),
todosList,
new HorizontalLayout(
taskField,
addButton
)
);
}
}
-
This
@Route
annotation makes the view accessible to the end user, in this case, using the empty `` route. -
As the the
MainView
class extendsVerticalLayout
, components added to it will be ordered vertically. -
todosList
is a vertical layout that displays a list of the tasks along with checkboxes. -
taskField
is a text input field to enter the description of new tasks. -
addButton
is a button for adding a new task. -
In the listener for the button click, first create a new checkbox with the value from the
taskField
as its label, then add the checkbox to thetodosList
. -
Add a shortcut for the
addButton
component when the key is pressed. -
Call
add()
on theVerticalLayout
to display the components vertically. Notice thattaskField
andaddButton
are in aHorizontalLayout
, which puts them next to each other.
Step 3: Run the Application
To run the project in your IDE, launch Application.java
, which is located under src/main/java/org/vaadin/example
.
Alternatively, you can run the project from the command line by typing mvnw
(on Windows), or ./mvnw
(on macOS or Linux).
Then, in your browser, open localhost:8080
and you should see the following:
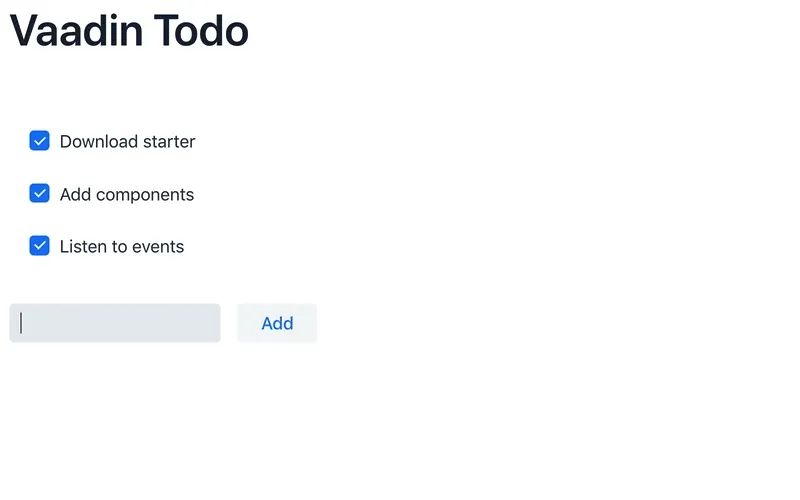
Go further
Now you have a taste of how Vaadin Flow empowers you to quickly build web apps in pure Java, without writing any HTML or JavaScript.
Continue exploring Vaadin Flow in the documentation, tutorials, and video courses:
Source code of the todo project is available on GitHub.
B4DF08AB-8BE6-4CC1-B8AF-B47FA07C3795