Payment Request API is a new, streamlined way to collect payment and shipping details from the user. Its main goal is to eliminate checkout forms completely by standardizing the payment communication flow as much as possible. Some benefits of using Payment Request API over traditional checkout forms are:
- Platform native and familiar for the user
- If the user gives consent, the payment data can be synced between desktop and mobile
- It’s simple to use from the developers point of view
For developers, collecting the payment details using Payment Request API couldn’t be more simple. Just create a request using the PaymentRequest
constructor, and call the object’s show()
method:
const supportedPaymentMethods = [
{
supportedMethods: 'basic-card',
}
];
const paymentDetails = {
total: {
label: 'Total',
amount:{
currency: 'USD',
value: 0
}
}
};
const request = new PaymentRequest(
supportedPaymentMethods,
paymentDetails
);
request.show()
.then(...).catch(...);
The constructor has two mandatory arguments: supported methods of payment and payment details. The show method returns a promise which will be fulfilled with the payment data once the user has authorized the payment.
Using in Vaadin 10 front-end applications
Using Payment Request API directly from JavaScript is really simple. Here’s a simple front-end demo application using the Vaadin components and Polymer. You can also test the demo online.
This is what the payment request looks like for the end-user in Chrome on mobile and desktop:
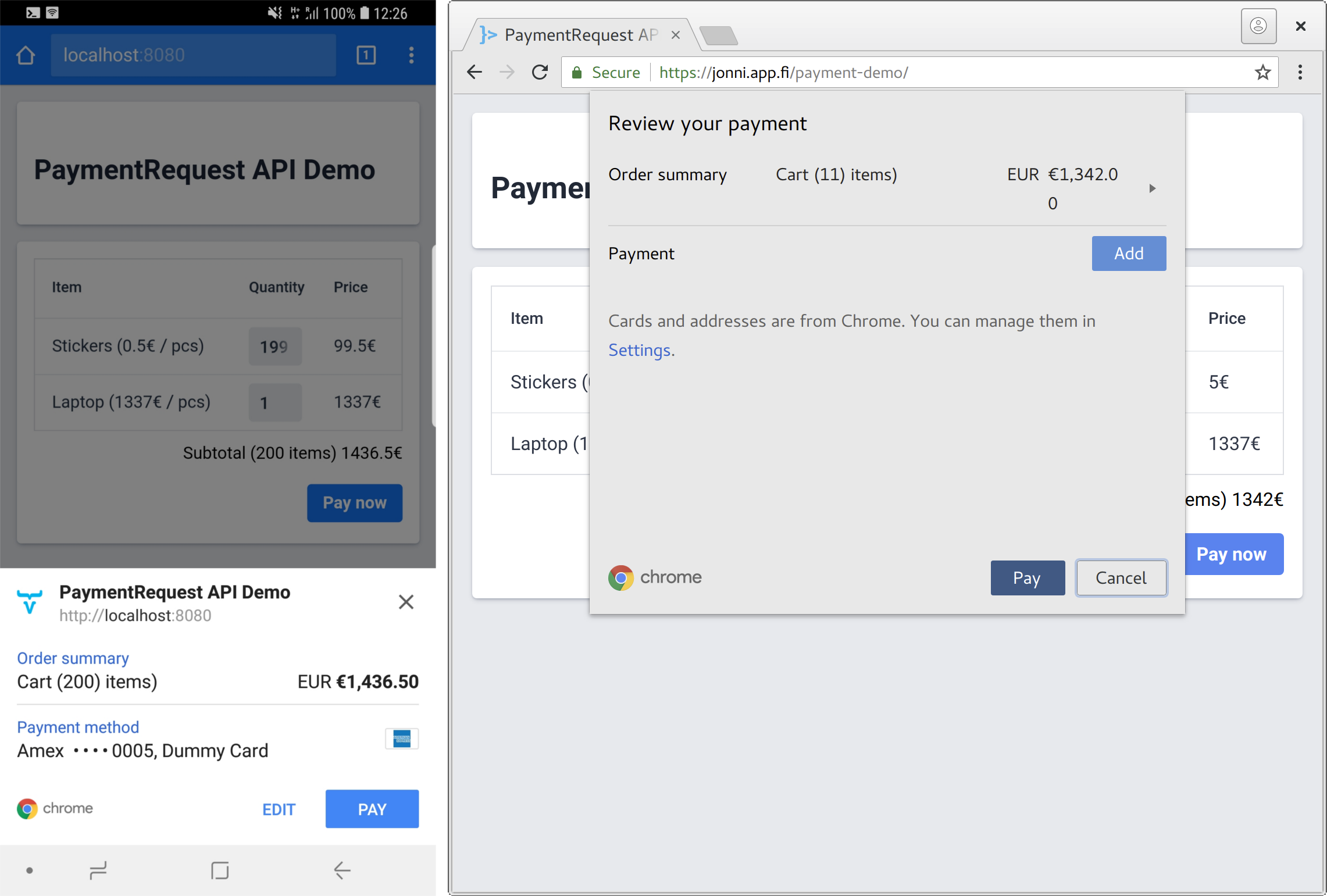
Vaadin 10 addon
To use the Payment Request API more easily from server-side Vaadin 10 Java code, I also created a Proof-of-Concept Vaadin 10 Payment Request Addon. The addon's test UI is deployed as an online demo. The Payment Request Addon provides a Java API to initiate the Payment Request and read the payment details, billing address etc. that the user has inputted.
With the add-on you create a payment request similarly to how you would use it in JavaScript:
PaymentRequest paymentRequest = new PaymentRequest(supportedPaymentMethods, paymentDetails);
But because the PaymentRequest.show()
method must be called from within a secure context, the installation of the event listener is a bit different from how you would normally react to user actions in Vaadin with ClickListeners
etc. Instead, you can install the PaymentRequest
into any Vaadin Component as a client-side click listener:
Button button = new Button("Pay now");
paymentRequest.install(button);
The last thing is to set a callback for the PaymentResponse:
paymentRequest.setPaymentResponseCallback((paymentResponse) -> {...}
You can access the user inputted payment details via PaymentResponse's getEventData(), e.g.
JsonObject eventData = paymentResponse.getEventData();
eventData.getObject("details").getString("cardNumber")
The add-on is still in early stages of development. It lacks major features, like shipping address collection, server-side notification on cancellation etc. Please let us know in the comments if you would have a need for a more complete way of handling payments with the Payment Request API.
Download the addon from Vaadin Directory