Vaadin 14 is the latest Long-term support (LTS) version of the Java web framework. Since the previous LTS version (Vaadin 10), we have released a lot of great features, like CDI support, dynamic route registration, Material theme, keyboard shortcuts, and 18 new components, to name a few. Assuming you have been following the releases during the last year, let’s focus on the top 14 new features introduced in Vaadin 14 in the past few months.
#1 Grid now supports automatic column sizing
Vaadin Grid now supports the automatic sizing of columns. By default, columns are sized equally. By setting the auto-width property to true on a column, it will attempt to fit its contents.
grid.getColumns().forEach(column -> column.setAutoWidth(true));

#2 Drag and drop rows in Grid
Drag and drop support was added to Vaadin Grid. On the source grid, enable dragging and define what data should get transferred. On the receiving grid, define how the dropped data should be handled. Both single and multi-row dragging is supported. Individual rows can be excluded from dnd operations by using drag and drop filters.
grid1.setRowsDraggable(true);
grid1.setDragDataGenerator("text/email", Person::getEmail);
Grid<Person> grid2 = new Grid<>(Person.class);
grid2.setDropMode(GridDropMode.BETWEEN);
grid2.addDropListener(event -> { /* ... */ } );
#3 Custom renderers in Grid Pro
Grid Pro now allows you to customize both the rendered values and components used for editing.
Use ComponentRenderer for customizing how the value is displayed.
ComponentRenderer<Span, Person> booleanRenderer = new ComponentRenderer<>(person -> new Span(person.isSubscriber() ? "Yes" : "No"));
grid1.addEditColumn(Person::isSubscriber, booleanRenderer).checkbox(Person::setSubscriber).setHeader("Subscriber");
For customizing the editor, you can now do it with the `custom` method when configuring an edit column.
Input customInput = new Input();
grid1.addEditColumn(Person::getEmail).custom(customInput, Person::setEmail).setHeader("Email");
#4 Notification now has theme variants
The Notification component has new theme variants to better convey their meaning. The new variants are primary, contrast, success, and error.
primary.addThemeVariants(NotificationVariant.LUMO_PRIMARY);
contrast.addThemeVariants(NotificationVariant.LUMO_CONTRAST);
success.addThemeVariants(NotificationVariant.LUMO_SUCCESS);
error.addThemeVariants(NotificationVariant.LUMO_ERROR);
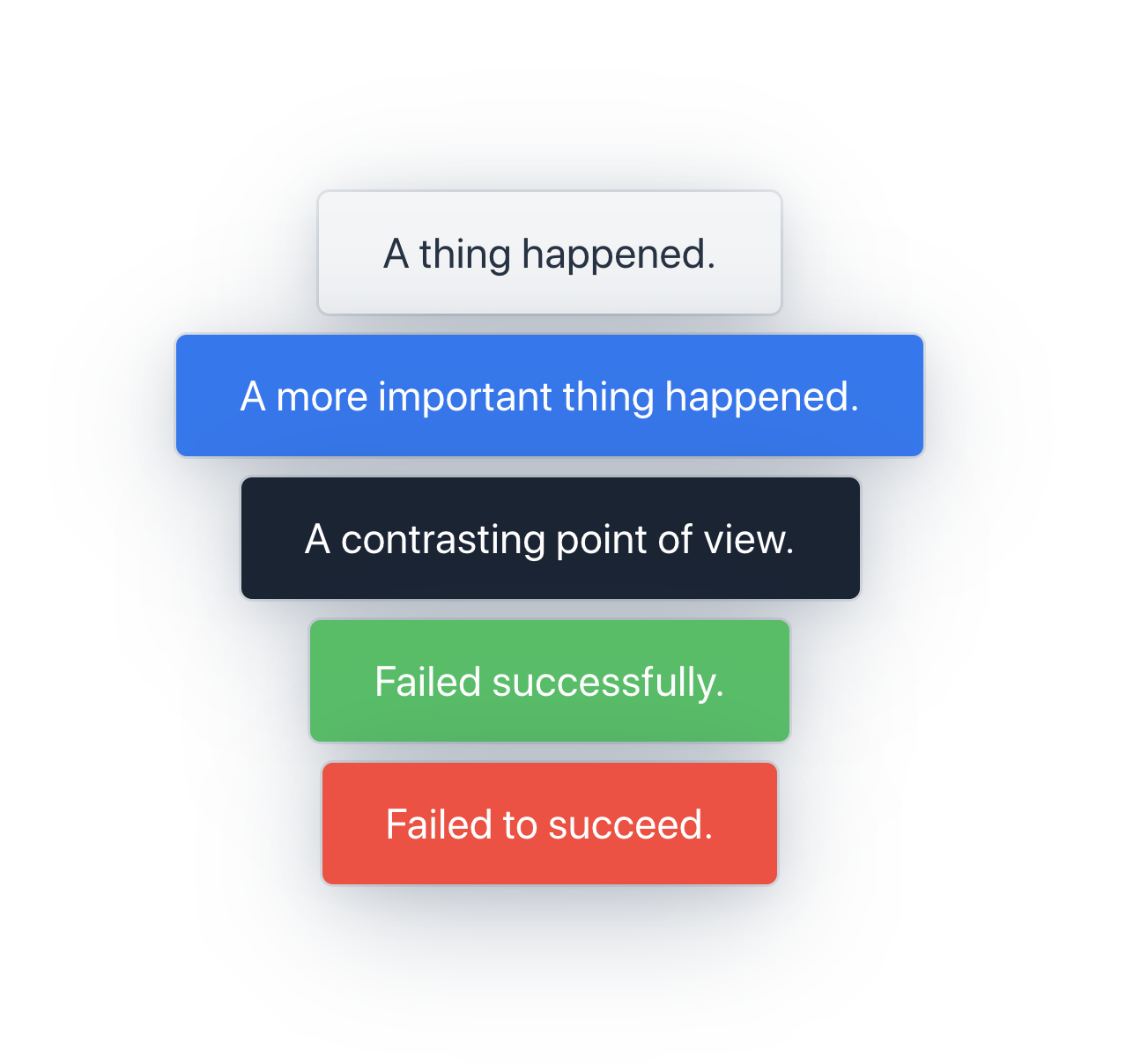
#5 Lazy value change events from Text Field
There are two new timeout modes for value changes on inputs. The previous eager mode fired on every keystroke which could be too much for some use cases. The lazy mode waits for the user to stop typing for a while before triggering. The timeout mode fires at configurable intervals, as long as there are updates.
textField.setValueChangeMode(ValueChangeMode.LAZY);
textField.setValueChangeMode(ValueChangeMode.TIMEOUT);
#6 PreserveOnRefresh allows keeping changes between page refreshes
Vaadin 14 brings back the PreserveOnRefresh annotation that was present in earlier Vaadin versions. Use it on a route to return the same instance of the view, instead of new instances, whenever you refresh the page.
#7 Possibility to hide the input field clear button
In Vaadin versions 10 to 13, the clear button was visible by default on inputs with a value. In Vaadin 14, the button is hidden by default. You can toggle the visibility with
setClearButtonVisible(boolean);
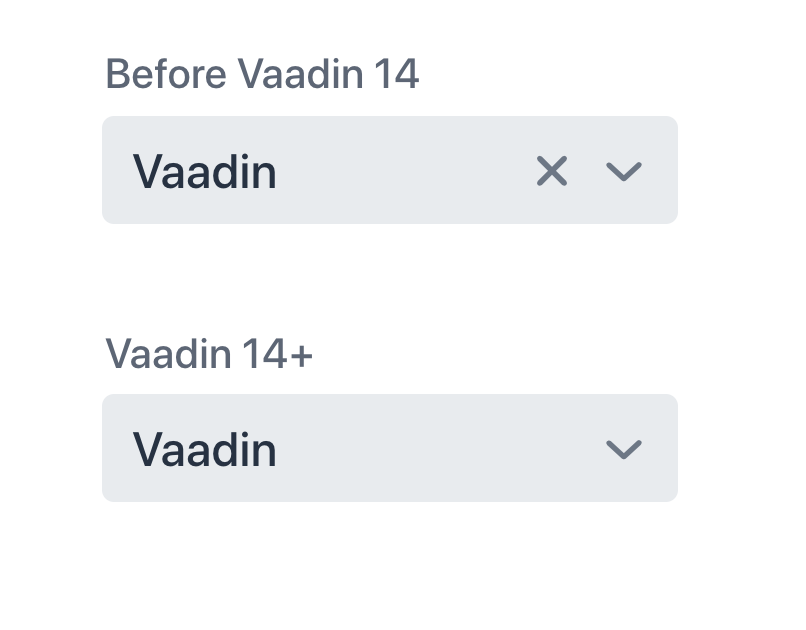
#8 Responsive side menu for App Layout
Vaadin 14 ships with a new version of App Layout. Now you can create left side navigation, in addition to the top navigation previously available in App Layout. The side navigation can be hidden by the user with a drawer toggle.
public class MainLayout extends AppLayout {
public MainLayout() {
final DrawerToggle drawerToggle = new DrawerToggle();
final RouterLink home = new RouterLink("Main", MainView.class);
final RouterLink about = new RouterLink("About", AboutView.class);
final VerticalLayout menuLayout = new VerticalLayout(home, about);
addToDrawer(menuLayout);
addToNavbar(drawerToggle);
}
}
@Route( layout = MainLayout.class)
public class MainView extends VerticalLayout {
}
@Route(layout = MainLayout.class)
public class AboutView extends VerticalLayout {
}
#9 Menu Bar component
The new Menu Bar component enables you to build dropdown menus with submenus. Menus are created by defining menu items and submenus for them.
MenuBar menuBar = new MenuBar();
MenuItem account = menuBar.addItem("Account");
MenuItem project = menuBar.addItem("Project");
SubMenu projectSubMenu = project.getSubMenu();
MenuItem user = projectSubMenu.addItem("Users");
MenuItem billing = projectSubMenu.addItem("Billing");
#10 Support for npm and ES6 modules
One of the biggest changes in Vaadin 14 is under the hood. Instead of using Bower for frontend dependencies, it now uses the industry-standard npm package manager and webpack build tool.
Templates are now defined as JavaScript files. This makes it easy to integrate 3rd party components, like Google’s model viewer here. On the server-side, load the template with @JsModule annotation and you’re good to go.
import { PolymerElement } from ‘@polymer/polymer/polymer-element.js’;
import { html } from ‘@polymer/polymer/lib/utils/html-tag.js’;
import '@google/model-viewer';
class TemplateView extends PolymerElement {
static get template() {
return html`
<model-viewer src="astronaut.glb" camera-controls auto-rotate></model-viewer>
`;
}
}
customElements.define("template-view", TemplateView);
@Route("template")
@Tag("template-view")
@JsModule("./src/template-view.js")
public class TemplateView extends PolymerTemplate<TemplateView.Model> {
public interface Model extends TemplateModel {}
}
#11 JavaScript calls from server-side can return values
Interacting with JavaScript is now easier, as you can get return values from executing JavaScript on the server-side. The executeJs method allows you to specify a callback function and the return data type so you can handle it on the server.
getElement()
.executeJs("return 'serviceWorker' in navigator")
.then(Boolean.class, supported -> {
if (supported) {
add(new Span("ServiceWorker is supported!"));
} else {
add(new Span("ServiceWorker is not supported!"));
}
});
#12 Server-side code receives detailed browser info
It's now possible to read client details, like time zone setting and screen resolution, on the server-side code using the browser details API.
UI.getCurrent()
.getPage()
.retrieveExtendedClientDetails(details -> {
add(new Span("Client resolution: "));
add(new Span(details.getScreenWidth() + "x" + details.getScreenHeight()));
});
#13 Embedding Vaadin components as web components
As of Vaadin 14, you are able to export your Vaadin components to be re-used as web components in other environments. This can be very handy when converting projects from other frameworks or integrating with legacy systems.
Start out by defining an exporter for the component you are exporting. Give it an HTML tag name that includes a dash.
public class MyComponentExporter extends WebComponentExporter<MyComponent> {
public MyComponentExporter() {
super("my-component");
}
@Override
protected void configureInstance(
WebComponent<MyComponent> webComponent,
MyComponent component) {
// Any custom initialization here
}
}
When you build the application, Vaadin will turn the exported component into a JavaScript file that you can include in a web page.
#14 Vaadin Plug-in for Eclipse for creating a new Vaadin project
There is now a new way to create a new Vaadin project in Eclipse. Find the Vaadin plug-in from Eclipse Marketplace and install the plug-in. You can create a project based on Spring Boot, Java EE, or plain Java servlet.
Try out Vaadin 14 today
Vaadin 14 is a long-term support (LTS) release, which means it will receive a full five years of free maintenance, and an additional ten years for Enterprise subscription customers. Head to vaadin.com/start where you can find the various ways in which to get started with Vaadin. On the start page, there is also a quick start tutorial which we recommend, if you are new to Vaadin.