What is new in Vaadin 17
Here are the highlights of the new and improved features in Vaadin 17.
The latest stable release of Vaadin 17 is 17.0.11, published on Nov 30, 2020. You can find all the release notes of the Vaadin platform in GitHub.
Improved data-binding Java API for Grid
Query size is now optional for lazy data binding. If you omit the item count, Grid polls your backend for more when the user scrolls to the end of the table. Learn about lazy data binding in the docs.
Vaadin 17 simplifies implementing Grid data binding to Spring Data repositories and other backends that use “paged access”. The Grid.setPageSize method is now guaranteed to affect the queries made to your data-binding callbacks. There is also a helper to get the page index, instead of forcing you to calculate it yourself.
// New Query.getPage() and Query.getPageSize() simplify creating page request
// Converting Vaadin data provider SortDirection
// to org.springframework.data.domain.Sort is straightforward with VaadinSpringDataHelpers
grid.setItems(q -> repo.findAll(PageRequest.of(q.getPage(), q.getPageSize(),
VaadinSpringDataHelpers.toSpringDataSort(q)));
A new dataview
API helps in common in-memory data-binding use cases. For example, it is now easier to mutate an existing in-memory data set or find the index of a specified item in the Grid.
import com.vaadin.flow.component.button.Button;
import com.vaadin.flow.component.grid.Grid;
import com.vaadin.flow.component.grid.dataview.GridListDataView;
import com.vaadin.flow.component.notification.Notification;
import com.vaadin.flow.component.textfield.TextField;
GridListDataView<Person> dataView = grid.setItems(myItems);
TextField newItemField = new TextField("Add new item");
Button addNewItem = new Button("Add", e -> {
// this adds new item to the backing list and
// automatically refreshes the component accordingly
dataView.addItem(new Person(newItemField.getValue()));
});
Button remove = new Button("Remove selected", e -> {
// remove item without resetting the options
dataView.removeItem(grid.asSingleSelect().getValue());
});
Button selectNextItem = new Button("Select next", e -> {
// Get current value, detect and select next
Person currentValue = grid.asSingleSelect().getValue();
dataView.getNextItem(currentValue).ifPresent(next -> grid.select(next));
});
// Subscribe to an event to be notified when the amount
// of items in the component changes
dataView.addItemCountChangeListener(e -> {
Notification.show(" " + e.getItemCount() + " items available");
});
// Add your custom code to export the items from the stream
Stream<Person> currentlyShownItems = dataView.getItems();
Form-binding API for client-side views
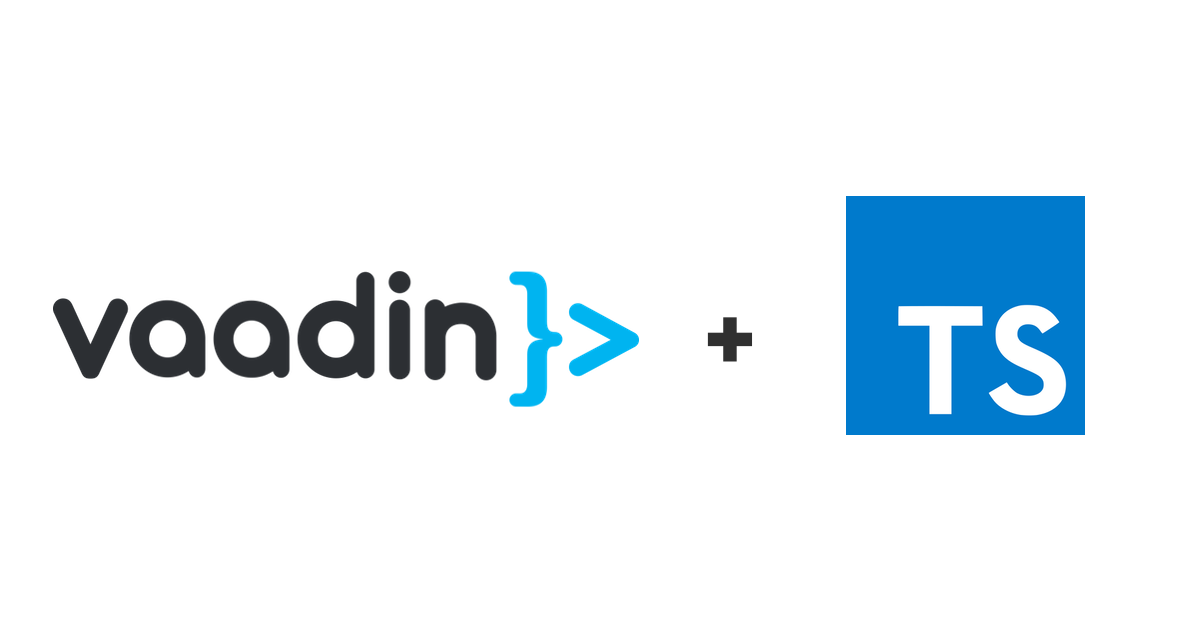
There are new improvements to the way you create forms for developers who prefer coding with TypeScript. A new client-side Binder supports Java-backend endpoints for loading and saving the form data, and reusing the metadata from Java Bean validation annotations for client-side validation.
// Employee.java
package com.example.application;
import javax.validation.constraints.Email;
import javax.validation.constraints.NotBlank;
public class Employee {
@Email(message = "Please enter a valid e-mail address")
@NotBlank
private String email;
// + other fields, constructors, setters and getters
}
// my-form-view.ts
import { Binder, field } from '@vaadin/form';
import EmployeeModel from './generated/com/example/application/EmployeeModel';
private binder = new Binder(this, EmployeeModel);
render() {
const { model } = this.binder;
return html`
<vaadin-email-field label="Email"
...=${field(model.email)}></vaadin-email-field>
`;
}
// Rest of the template code
Read the docs on how to build client-side forms using templates and the web component API in TypeScript.
TypeScript definitions for all Vaadin components
All Vaadin components now have TypeScript type definitions to improve working with TypeScript-based views in IDEs, for example for improved code completion and auto imports.
More chart types and a Java API to style charts
Charts for Vaadin 17 comes with four new chart types, and the Java API for styling makes a comeback. The underlying Highcharts library is updated to version 8.
The four new chart types include Bullet, Organization, Timeline and X Range.
Bullet
Compares one value to another and relates them to qualitative ranges.

X Range
Visualizes ranges on the X-axis. An X-range is similar to a column range, but displays X-data ranges, as opposed to the lows and highs in Y data.

Timeline
Visualizes important events or milestones over time.
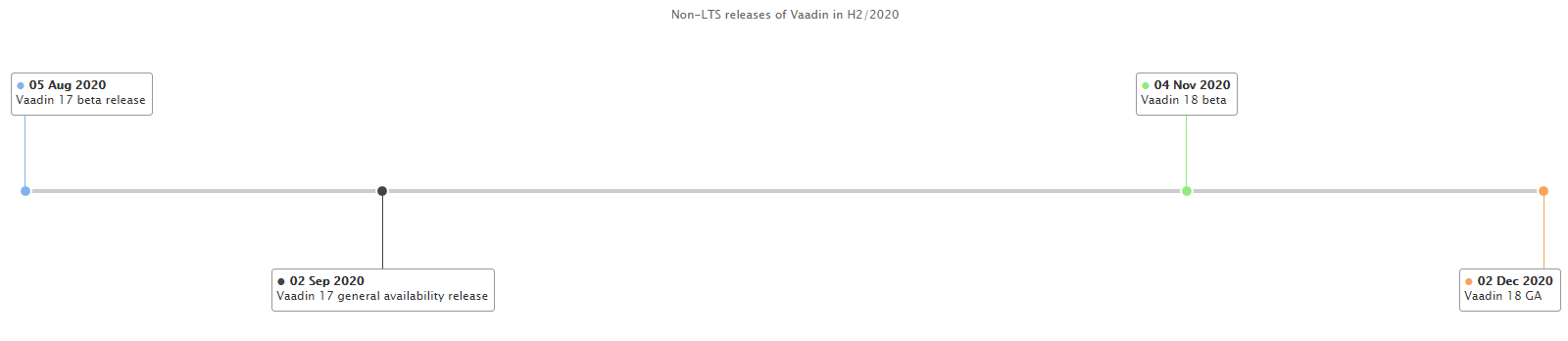
Organization
Visualizes the structure of an organization and its relationships and relative ranks.
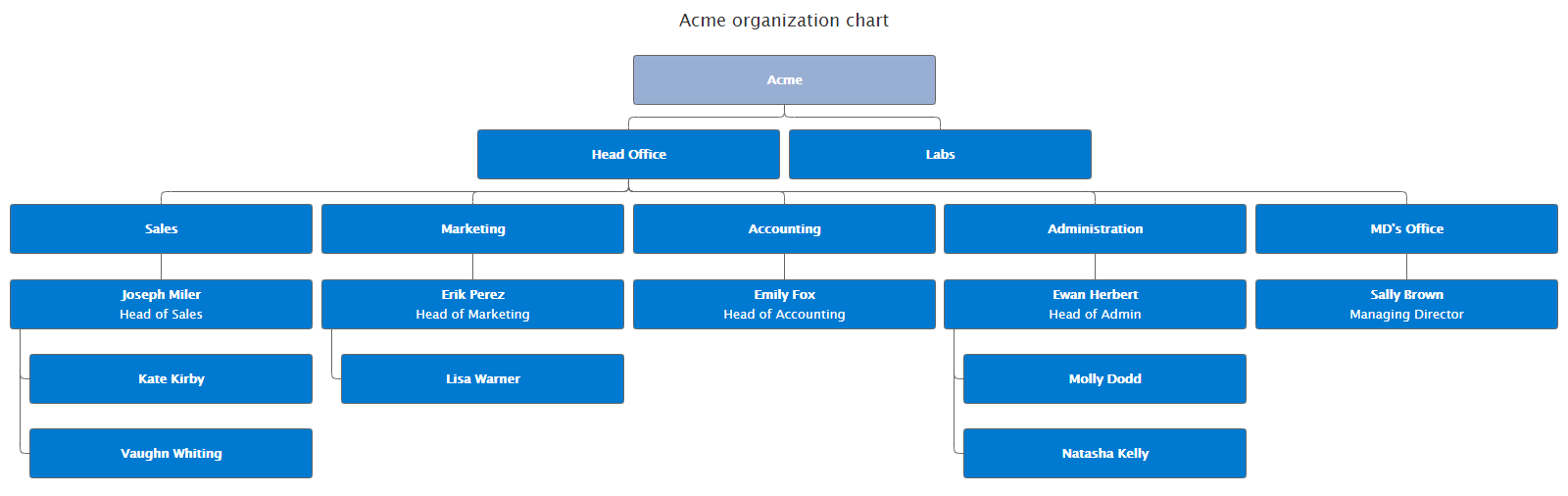
Charts styling Java API
In Vaadin 10, we dropped the Java API for styling charts in favor of CSS styling. Its return became a much-requested feature and we are now bringing it back as the new default. It comes in handy, especially for developers migrating Vaadin 8 code. CSS styling is still available through a configuration flag.
// Example of setting y-axis label styling
YAxis yaxis = new YAxis();
Labels ylabels = yaxis.getLabels();
ylabels.getStyle().setColor(new SolidColor("#4F5B67"));
ylabels.getStyle().setFontWeight(FontWeight.BOLD);
conf.addyAxis(yaxis);
LitTemplate for declarative server-side views
You can create server-side views programmatically or declaratively. For declarative views, it’s now possible to use LitElement instead of Polymer for view templates. Polymer templates are still supported in the toolkit. When creating a LitElement template for a server-side view, you should combine it with Java UI logic. Write static HTML for the layout and use the @Id annotation to bind the components to server-side component instances. Currently, you need to create the LitElement templates by hand, but support for Vaadin Designer is coming.
// <project-root>/frontend/my-lit-view.ts
import {LitElement, html} from 'lit-element';
import '@vaadin/vaadin-button/vaadin-button';
import '@vaadin/vaadin-text-field/vaadin-text-field';
import '@vaadin/vaadin-ordered-layout/vaadin-vertical-layout';
class MyLitView extends LitElement {
render() {
return html`
<vaadin-vertical-layout theme="margin spacing">
<p>A view created with layout from
a LitElement template and logic in server-side Java code.</p>
<vaadin-text-field label="Name" id="nameField"></vaadin-text-field>
<vaadin-button id="helloButton">Say Hello</vaadin-button>
</vaadin-vertical-layout>`;
}
}
customElements.define(my-lit-view, MyLitView);
// MyLitView.java
import com.vaadin.flow.component.Tag;
import com.vaadin.flow.component.button.Button;
import com.vaadin.flow.component.dependency.JsModule;
import com.vaadin.flow.component.littemplate.LitTemplate;
import com.vaadin.flow.component.notification.Notification;
import com.vaadin.flow.component.polymertemplate.Id;
import com.vaadin.flow.component.textfield.TextField;
import com.vaadin.flow.router.Route;
@Route("/myview")
@Tag("my-lit-view")
@JsModule("./my-lit-view.ts")
public class MyLitView extends LitTemplate {
@Id
private Button helloButton;
@Id
private TextField nameField;
public MyLitView() {
helloButton.addClickListener(event -> Notification.show("Hello " + nameField.getValue()));
}
}
See the docs for more.
Route templates for more flexible routing
Routing in server-side views now supports more complex use cases. Read the docs to see how you can define routes with optional parameters, wildcard parameters and routes matching a given regular expression.
Improved live reload with Java hot-swap tools
Vaadin 17 includes improvements to the live reload feature introduced in Vaadin 14.3 LTS.
Update instructions
- Upgrading from Vaadin 14?
- Upgrading from Vaadin 16?
- Just upgrade the version number in your pom.xml to
17.0.11
- Just upgrade the version number in your pom.xml to
Supported browsers and Java versions
- Latest versions of the following browsers:
- Edge Chromium
- Latest Firefox and the latest Firefox ESR
- Chrome
- Safari
- Supported Java versions:
- Java 8
- Java 11
- The latest Java
Vaadin 17 is supported until January 2021
Vaadin 17 is a non-LTS release and is maintained until one month after version 18.0 is released in December 2020. Maintenance guarantees start from the general availability release (GA). Vaadin 14 is the current long-term support (LTS) version and is the recommended version for most users.