Nested Rows
While building your board, sometimes you might want to add more components into one row. To do that, Vaadin Board support nested regions so you can add a row inside another one and let Vaadin Board handle the work, thanks to its responsiveness capabilities.
Here, we are going to show how to create nested rows and how they will help you. This chapter assumes you have already added Vaadin Board to your project and installed the license key. If not, check the instructions in "Getting Started Section".
Basic Usage
For this example, we are going to create a row with 2 buttons and place it inside a outer row.
protected void init(VaadinRequest request) {
VerticalLayout container = new VerticalLayout();
Board board = new Board();
Button button1 = new Button("Button 1");
Button button2 = new Button("Button 2");
Button button3 = new Button("Button 3");
Row outerRow = board.addRow(button1, button2, button3);
Row nestedRow = new Row();
Button inner1 = new Button("Inner 1");
Button inner2 = new Button("Inner 2");
nestedRow.addComponents(inner1, inner2);
outerRow.addNestedRow(nestedRow);
container.addComponent(board);
setContent(container);
}
The result for different view port is the following:


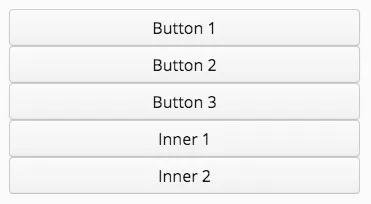
Also, you can opt to add nestedRow
to outerRow
either by calling outerRow.addComponent(nestedRow)
(this is possible because Row
is also a Component
) or at board.addRow(button1, button2, button3, nestedRow)
.
Component Span
As any other component inside a row, the nested row can also be spanned to fill more than one column (keep in mind that the maximum number of columns for each row is 4). Take this example, based on the previous one:
protected void init(VaadinRequest request) {
VerticalLayout container = new VerticalLayout();
container.setMargin(true);
container.setSizeFull();
Board board = new Board();
Button button1 = new Button("Button 1");
Button button2 = new Button("Button 2");
Row outerRow = board.addRow(button1, button2);
Row nestedRow = new Row();
Button inner1 = new Button("Inner 1");
Button inner2 = new Button("Inner 2");
Button inner3 = new Button("Inner 3");
Button inner4 = new Button("Inner 4");
nestedRow.addComponents(inner1, inner2, inner3, inner4);
outerRow.addNestedRow(nestedRow);
outerRow.setComponentSpan(nestedRow, 2);
container.addComponent(board);
setContent(container);
}
We are telling that nestedRow
will fill 2 columns of the row. This example will produce the following result for each view port:


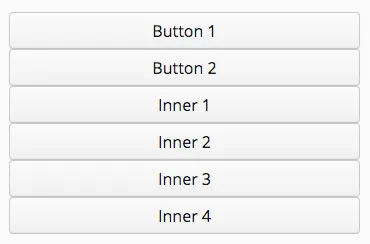