Sorry when i must come again.
But it still not works.
I try to explain you what is my problem.
I am using Java EE7 + Wildfly 11 + Vaadin 8.
All should be managed by the DI Container.
I want to design a Application like in the picture attached.
My Problem is to realize the navigation in Part 2.
In “Part 1” I managed my Navigation so:
When i click the Button Address then all Addresses from the database are shown in the Content01View-Area.
When i click the Button Help then Help-Text is shown in the Content01View-Area.
All goes well.
I use the Source-Code of the following 2 classes:
package org.app.ui;
@CDIUI("")
@PushStateNavigation
public class MainView extends UI {
@Inject
CDIViewProvider viewProvider;
private Navigator navigator;
public static final String PERSON_VIEW = "Person";
public static final String MASTER_DETAIL_VIEW = "MasterDetail";
public static final String HELP_VIEW = "Help";
@Override
protected void init(VaadinRequest request) {
final VerticalLayout mainLayout = new VerticalLayout();
final CssLayout menuView = new CssLayout();
final CssLayout content01View = new CssLayout();
mainLayout.addComponent(menuView);
mainLayout.addComponent(content01View);
mainLayout.setMargin(true);
mainLayout.setSpacing(true);
setContent(mainLayout);
navigator = new Navigator(this, content01View);
navigator.addView("", new LoginView());
navigator.addView(PERSON_VIEW, new PersonView());
navigator.addView(MASTER_DETAIL_VIEW, new MasterDetailView());
navigator.addView(HELP_VIEW, new HelpView());
}
}
package org.app.ui;
@PushStateNavigation
public class TopMenu extends CustomComponent {
public TopMenu() {
HorizontalLayout layout = new HorizontalLayout();
layout.addComponent(personViewButton);
layout.addComponent(masterDetailViewButton);
layout.addComponent(helpViewButton);
layout.addComponent(logoutButton());
layout.setSizeUndefined();
layout.setSpacing(true);
setSizeUndefined();
setCompositionRoot(layout);
}
Button personViewButton = new Button(MainView.PERSON_VIEW,
e -> UI.getCurrent().getNavigator().navigateTo(MainView.PERSON_VIEW));
Button masterDetailViewButton = new Button("Master Detail",
e -> UI.getCurrent().getNavigator().navigateTo(MainView.MASTER_DETAIL_VIEW));
Button helpViewButton = new Button(MainView.HELP_VIEW,
e -> UI.getCurrent().getNavigator().navigateTo(MainView.HELP_VIEW));
}
I navigate now in Part 1 to MasterDetailView. This works fine.
I get Problems now to navigate in Part 2, when i want to navigate in the MasterDetailView to the TitleView.
package org.app.ui.masterdetail;
@SuppressWarnings("serial")
@CDIView("")
public class MasterDetailView extends VerticalLayout implements View {
@Inject
TitleService service;
private TopMenu topMenu;
private HorizontalLayout allView;
private CssLayout leftMenu;
private CssLayout content02View;
private Button title;
public MasterDetailView() {
topMenu = new TopMenu();
allView = new HorizontalLayout();
leftMenu = new CssLayout();
leftMenu.addStyleName(ValoTheme.MENU_ROOT);
content02View = new CssLayout();
Label cLabel = new Label("ContentView");
Label head = new Label("LeftMenu");
head.addStyleName(ValoTheme.MENU_TITLE);
leftMenu.addComponent(head);
leftMenu.addComponent(showTitleView());
content02View.addComponent(cLabel);
allView.addComponent(leftMenu);
allView.addComponent(content02View);
allView.setSizeFull();
addComponent(topMenu);
addComponent(allView);
title.addClickListener(event ->
title.setCaption("You pushed it!"));
}
private Button showTitleView() {
title = new Button("Title", new Button.ClickListener() {
@Override
public void buttonClick(ClickEvent event) {
content02View.removeAllComponents();
content02View.addComponent(new TitleView(service));
}
});
return title;
}
}
package org.app.ui.masterdetail.views;
public class TitleView extends VerticalLayout implements View {
public TitleView(TitleService service) {
Label label01 = new Label("List1 of Title");
addComponent(label01);
Label label02 = new Label(service.getTitle(2).getTitle());
addComponent(label02);
}
}
I tried to make it so, but it doesn’t work.
My Question:
How can i show in my Case the content of TitleView in the area of Content02View?
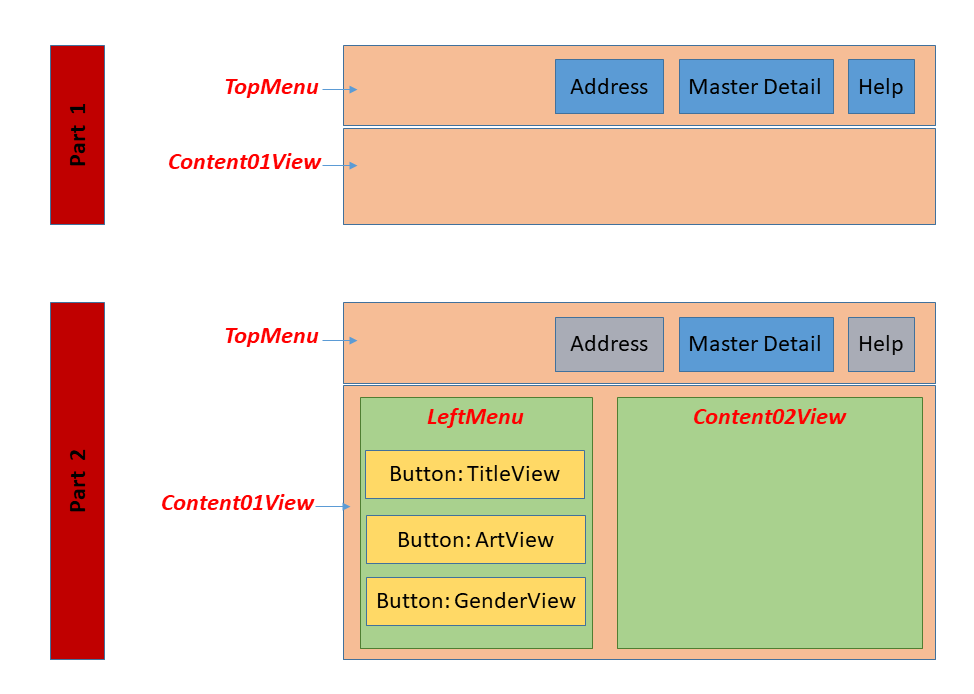