Hi i am trying to do as follows.But not able to see the data from all three maps
LinkedHashMap<String, Object> h1 = new LinkedHashMap<>();
h1.put(“C1”, “Test11”);
h1.put(“C2”, “Test12”);
h1.put(“C3”, new Date());
LinkedHashMap<String, Object> h2 = new LinkedHashMap<>();
h2.put(“C1”, “Test21”);
h2.put(“C2”, “Test22”);
h2.put(“C3”, new Date());
LinkedHashMap<String, Object> h3 = new LinkedHashMap<>();
h3.put(“C1”, “Test31”);
h3.put(“C2”, “Test32”);
h3.put(“C3”, new Date());
List<HashMap<String, Object>> allrows = new ArrayList<>();
allrows.add(h1);
allrows.add(h2);
allrows.add(h3);
Grid<HashMap<String, Object>> grid = new Grid<>();
HashMap<String, Object> s = allrows.get(0);
for (Map.Entry<String, Object> entry : s.entrySet()) {
grid.addColumn(h → s.get(entry.getKey())).setCaption(entry.getKey());
}
grid.setItems(allrows);
grid.select(allrows.get(0));
I am getting data only from h1 map not from h2 and h3.
Hear i attached the screen shot .
Issue 2:
And I am trying to set the Visible columns using
String pGridColumns1={“C1”,“C2”};
grid.setColumns(pGridColumns);
I Am getting below exception
java.lang.IllegalStateException: A Grid created without a bean type class literal or a custom property set doesn’t support finding properties by name.
at com.vaadin.ui.Grid$1.getProperty(Grid.java:2304)
Regards
Nagaraj RC
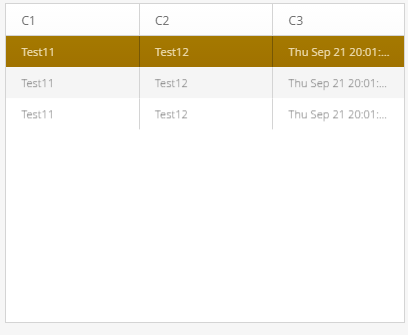