Multiselect Combo Box - Vaadin Add-on Directory
A multiselection component where items are displayed in a drop-down list.Issue tracker
Source Code
Multiselect Combo Box version 1.0.1
This release introduces various updates that enable the `multiselect-combo-box` web component to work in IE 11.
Multiselect Combo Box version 2.0.0
This is the first major release of the `MultiselectComboBox` that supports Vaadin 14 in `npm` mode and brings the same functionality to the latest version of the Vaadin platform. This release is based on version [`2.0.2`](https://github.com/gatanaso/multiselect-combo-box/releases/tag/v2.0.2) of the `multiselect-combo-box` web component.
To use this component with Vaadin 14 in `bower` mode, please use the latest `1.x.x` version.
Multiselect Combo Box version 2.1.0
This is the first version the `MultiselectComboBox` that supports lazy data loading. It adds a new API to support item filtering (fixes issue https://github.com/gatanaso/multiselect-combo-box-flow/issues/13) and furthermore, asynchronous / lazy loading of data items from the backend (fixes issue https://github.com/gatanaso/multiselect-combo-box-flow/issues/7)
Multiselect Combo Box version 2.2.0
To better align with the new defaults for the vaadin components, the `clear-button-visible` attribute is now also available to the `multiselect-combo-box`. With this update the component no longer displays the clear icon by default, but instead it needs to be specified explicitly. This change breaks the default behavior in favor of aligning better with the current default behavior of the `vaadin-text-field` and the `vaadin-combo-box` components.
⚠️ Breaking Change: The clear button is now hidden by default. To make it visible, set the `clearButtonVisible` property.
ℹ️ Note: if the value of the `multiselect-combo-box` is empty, the clear button is always hidden.
Multiselect Combo Box version 1.1.0
*release for the Vaadin 12/Vaadin 13 compatible version of this component*
To better align with the new defaults for the vaadin components, the `clear-button-visible` attribute is now also available to the `multiselect-combo-box`. With this update the component no longer displays the clear icon by default, but instead it needs to be specified explicitly. This change breaks the default behavior in favor of aligning better with the current default behavior of the `vaadin-text-field` and the `vaadin-combo-box` components.
⚠️ Breaking Change: The clear button is now hidden by default. To make it visible, set the `clearButtonVisible` property.
ℹ️ Note: if the value of the `multiselect-combo-box` is empty, the clear button is always hidden.
Multiselect Combo Box version 2.2.1
This is a bugfix release that contains the fixes for [#20](https://github.com/gatanaso/multiselect-combo-box-flow/issues/20) and [#21](https://github.com/gatanaso/multiselect-combo-box-flow/issues/21).
Multiselect Combo Box version 2.3.0
This release introduces a much needed feature of the `MultiselectComboBox` and that is to keep the overlay open when selecting items (this was not previously achievable due to limitations in the underlying `vaadin-combo-box`).
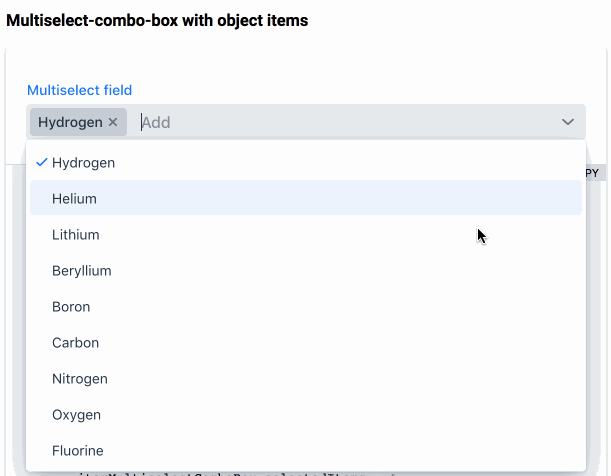
Furthermore, with this release the component no longer has a default full width, but instead, it takes the amount of space it needs. This change breaks previous behavior in favor of better aligning with the core set of vaadin components.
Also, a new property `readonlyValueSeparator` was introduced, which enables customizing the value separator that is used for constructing the display value when the component is in `readonly` mode.
The default value of this separator is `, `.
⚠️ Breaking change: the `MultiselectComboBox` no longer has a default full width.
Multiselect Combo Box version 2.3.1
This is a bugfix release that fixes issue [#30](https://github.com/gatanaso/multiselect-combo-box-flow/issues/30) and issue [#36](https://github.com/gatanaso/multiselect-combo-box-flow/issues/36).
Additionally, it updates the version of underlying `multiselect-combo-box` web component to [2.3.1](https://github.com/gatanaso/multiselect-combo-box/releases/tag/v2.3.1)
Multiselect Combo Box version 2.4.0
This release introduces the possibility to add custom values to the `MultiselectComboBox`!
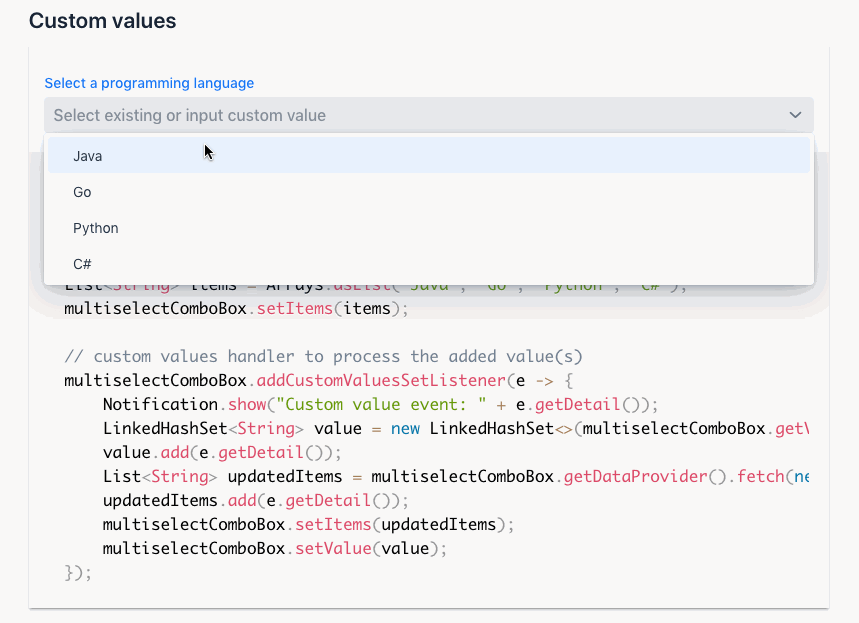
To enable custom values call `addCustomValuesSetListener`. It will add the listener for the event which is fired when user inputs a string value that does not match any existing items and commits it i.e. by pressing the enter-key. Note that `MultiselectComboBox` doesn't do anything with the custom value string automatically. Use this method to determine how the custom value should be handled.
For examples of how this works, check out the live [demo](https://multiselect-combo-box-flow.herokuapp.com/custom-values)
Multiselect Combo Box version 2.4.1
This is a bugfix release that fixes issue [#42](https://github.com/gatanaso/multiselect-combo-box-flow/issues/42). Additionally, it updates the version of underlying `multiselect-combo-box` web component to `2.4.1`.
Multiselect Combo Box version 3.0.0
This release adds support for Vaadin 15.
Multiselect Combo Box version 2.4.2
This is a bugfix release that fixes issues [#28](https://github.com/gatanaso/multiselect-combo-box-flow/issues/28), [#39](https://github.com/gatanaso/multiselect-combo-box-flow/issues/39) and [#46](https://github.com/gatanaso/multiselect-combo-box-flow/issues/46) which mainly revolve around using the `MultiselectComboBox` in a dialog or in a grid.
Additionally, it updates the version of underlying `multiselect-combo-box` web component to `2.4.2`
**This release targets Vaadin version 14**
Multiselect Combo Box version 3.0.1
This is a bugfix release that fixes issues [#28](https://github.com/gatanaso/multiselect-combo-box-flow/issues/28), [#39](https://github.com/gatanaso/multiselect-combo-box-flow/issues/39) and [#46](https://github.com/gatanaso/multiselect-combo-box-flow/issues/46) which mainly revolve around using the `MultiselectComboBox` in a dialog or in a grid.
Additionally, it updates the version of underlying `multiselect-combo-box` web component to `2.4.2`
**This release targets Vaadin version 15**
Multiselect Combo Box version 2.5.0
This release introduces the possibility to use `TemplateRenderer` and `ComponentRenderer` [#14](https://github.com/gatanaso/multiselect-combo-box-flow/issues/14) to customise individual items in the drop down list.
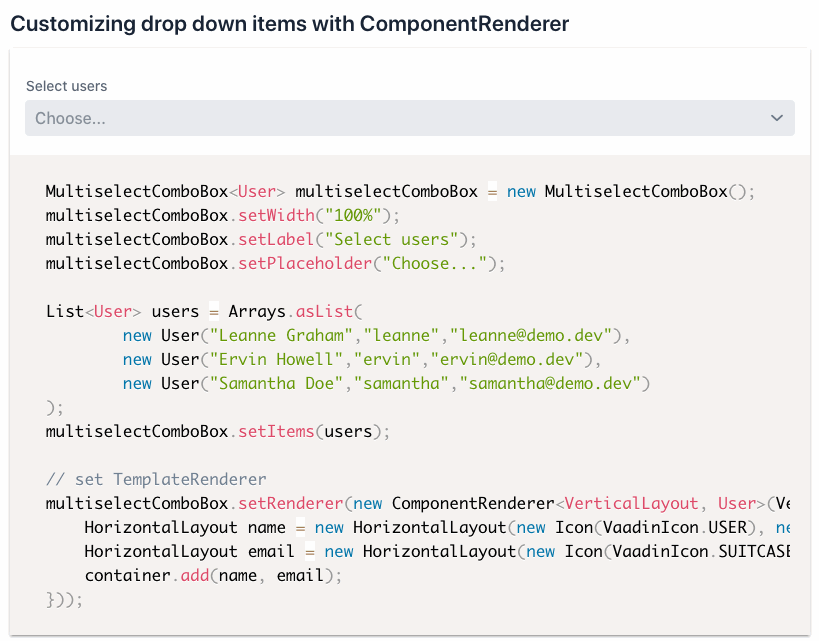
For examples of how to use this feature check out the [demo](https://multiselect-combo-box-flow.herokuapp.com/presentation).
ℹ️ This release targets **Vaadin 14**
Multiselect Combo Box version 3.0.2
This release introduces the possibility to use `TemplateRenderer` and `ComponentRenderer` [#14](https://github.com/gatanaso/multiselect-combo-box-flow/issues/14) to customise individual items in the drop down list.
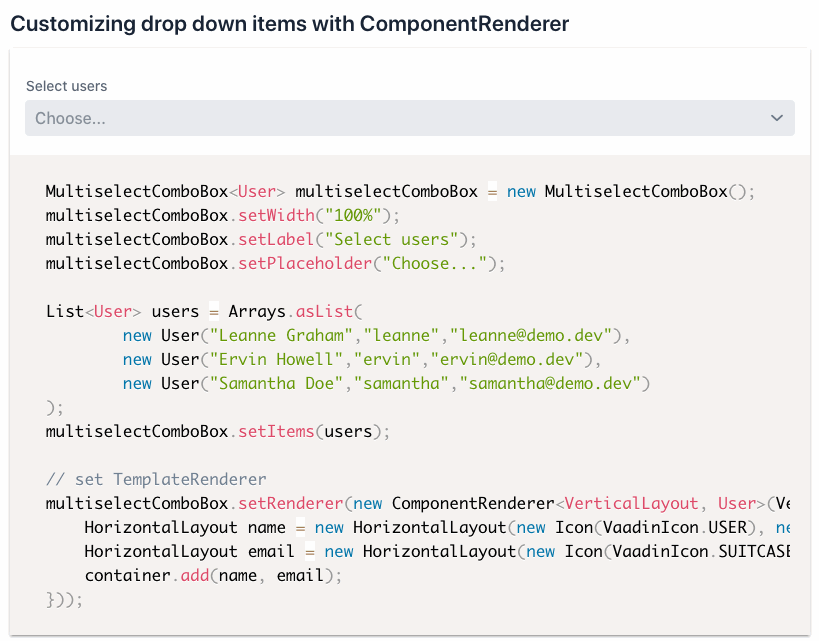
For examples of how to use this feature check out the [demo](https://multiselect-combo-box-flow.herokuapp.com/presentation).
ℹ️ This release targets **Vaadin 15** and **Vaadin 16**
Multiselect Combo Box version 4.0.0-rc2
This is the initial release candidate that provides support for Vaadin 22+.
Please note that this release uses the latest updated version of the web component (https://github.com/gatanaso/multiselect-combo-box/releases/tag/v3.0.0-alpha.2)