QCObjects - Vaadin Add-on Directory
Cross Browser Javascript Framework for MVC Patterns

QCObjects
=============
Cross Browser Javascript Framework for MVC Patterns
---------------------------------------------------
QCObjects is a javascript framework designed to make easier everything about the MVC patterns implementation into the pure javascript scope. You don't need to use typescript nor any transpiler to run QCObjects. It runs directly on the browser and it uses pure javascript with no extra dependencies of code. You can make your own components expressed in real native javascript objects or extend a native DOM object to use in your own way. You can also use QCObjects in conjunction with CSS3 frameworks like [Foundation] (https://foundation.zurb.com), [Bootstrap] (http://getbootstrap.com) and mobile javascript frameworks like [PhoneGap] (https://phonegap.com) and OnsenUI (https://onsen.io)

_________________________
Table of Contents
--------------------
- [Demo Using Foundation](#demo-using-foundation)
- [Demo Using Materializecss](#demo-using-materializecss)
- [Demo Using Raw CSS](#demo-using-raw-css)
- [Another Basic Demo example: The simpliest demo example:](#another-basic-demo-example-the-simpliest-demo-example)
- [Using QCObjects with Atom:](#using-qcobjects-with-atom)
- [Using QCObjects in Visual Studio Code:](#using-qcobjects-in-visual-studio-code)
- [Installing with NPM:](#installing-with-npm)
- [Using the code in the straight way into HTML5:](#using-the-code-in-the-straight-way-into-html5)
- [Quick Start](#quick-start)
- [Step 1: Start creating a main import file and name it like: cl.quickcorp.js. Put it into packages/js/ file directory](#step-1-start-creating-a-main-import-file-and-name-it-like-clquickcorpjs-put-it-into-packagesjs-file-directory)
- [Step 2: Then create some services inhereting classes into the file js/packages/cl.quickcorp.services.js :](#step-2-then-create-some-services-inhereting-classes-into-the-file-jspackagesclquickcorpservicesjs-)
- [Step 3: Now it's time to create the components (cl.quickcorp.components.js)](#step-3-now-its-time-to-create-the-components-clquickcorpcomponentsjs)
- [Step 4: Once you have done the above components declaration, you will now want to code your controllers (cl.quickcorp.controller.js)](#step-4-once-you-have-done-the-above-components-declaration-you-will-now-want-to-code-your-controllers-clquickcorpcontrollerjs)
Copyright
--------------
Copyright (c) Jean Machuca and QuickCorp
Demo
--------------
# Demo Using Foundation
Check out a demo using Foundation components here:
[Demo Using Foundation](https://github.com/QuickCorp/quickobjects_sample1foundation)
# Demo Using Materializecss
Check out a demo using MaterializeCSS here:
[Demo Using Materializecss](https://qln.link)
# Demo Using Raw CSS
Check out a demo using raw CSS:
[Demo Using Raw CSS](https://github.com/QuickCorp/qcobjects_profile_browser)
# Another Basic Demo example: The simpliest demo example:
```html
Demo
```
Fork
--------------
Please fork this project or make a link to this project into your README.md file. Read the LICENSE.txt file before you use this code.
Become a Sponsor
------------------
If you want to become a sponsor for this wonderful project you can do it [here](https://sponsorsignup.qcobjects.dev/)
Check out the QCObjects SDK
----------------------------
You can check out the [QCObjects SDK](https://sdk.qcobjects.dev/) and follow the examples to make your own featured components
Donate
--------------
If you like this code please [DONATE](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=UUTDBUQHCS4PU&source=url)!
[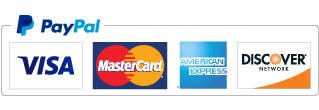](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=UUTDBUQHCS4PU&source=url)
Installing
------------
# Using QCObjects with Atom:
```shell
> apm install qcobjects-syntax
```
https://atom.io/packages/qcobjects-syntax
# Using QCObjects in Visual Studio Code:
https://marketplace.visualstudio.com/items?itemName=Quickcorp.QCObjects-vscode
# Installing with NPM:
```shell
> npm install qcobjects
```
# Using the code in the straight way into HTML5:
```html
```
# Quick Start
## Step 1: Start creating a main import file and name it like: cl.quickcorp.js. Put it into packages/js/ file directory
```javascript
"use strict";
/*
* QuickCorp/QCObjects is licensed under the
* GNU Lesser General Public License v3.0
* [LICENSE] (https://github.com/QuickCorp/QCObjects/blob/master/LICENSE.txt)
*
* Permissions of this copyleft license are conditioned on making available
* complete source code of licensed works and modifications under the same
* license or the GNU GPLv3. Copyright and license notices must be preserved.
* Contributors provide an express grant of patent rights. However, a larger
* work using the licensed work through interfaces provided by the licensed
* work may be distributed under different terms and without source code for
* the larger work.
*
* Copyright (C) 2015 Jean Machuca,
*
* Everyone is permitted to copy and distribute verbatim copies of this
* license document, but changing it is not allowed.
*/
Import ('external/libs');
Import ('cl.quickcorp.model');
Import ('cl.quickcorp.components');
Import ('cl.quickcorp.controller');
Import ('cl.quickcorp.view');
Package('cl.quickcorp',[
Class('FormValidator',Object,{
}),
]);
```
## Step 2: Then create some services inhereting classes into the file js/packages/cl.quickcorp.services.js :
```javascript
"use strict";
/*
* QuickCorp/QCObjects is licensed under the
* GNU Lesser General Public License v3.0
* [LICENSE] (https://github.com/QuickCorp/QCObjects/blob/master/LICENSE.txt)
*
* Permissions of this copyleft license are conditioned on making available
* complete source code of licensed works and modifications under the same
* license or the GNU GPLv3. Copyright and license notices must be preserved.
* Contributors provide an express grant of patent rights. However, a larger
* work using the licensed work through interfaces provided by the licensed
* work may be distributed under different terms and without source code for
* the larger work.
*
* Copyright (C) 2015 Jean Machuca,
*
* Everyone is permitted to copy and distribute verbatim copies of this
* license document, but changing it is not allowed.
*/
Package('cl.quickcorp.service',[
Class('JsonService',{
method:"GET",
cached:false,
headers: {
"Content-Type":"application/json",
"charset":"utf-8"
},
JSONresponse: null,
done:function(result){
console.log("***** RECEIVED RESPONSE:");
console.log(result.service.template);
this.JSONresponse = JSON.parse(result.service.template);
alert(this.template);
}
}),
Class('FormSubmitService',{
method:"POST",
cached:false,
headers: {
"Content-Type":"application/json"
},
JSONresponse: null,
done: function(result) {
console.log("***** CALLED FormSubmitService");
this.JSONresponse = JSON.parse(result.service.template);
//TODO success case
console.log("***** SUCCESS!")
console.log(this.JSONresponse);
},
fail: function(result) {
//TODO negative case
console.log("***** ERROR");
}
})
])
```
## Step 3: Now it's time to create the components (cl.quickcorp.components.js)
```javascript
"use strict";
/*
* QuickCorp/QCObjects is licensed under the
* GNU Lesser General Public License v3.0
* [LICENSE] (https://github.com/QuickCorp/QCObjects/blob/master/LICENSE.txt)
*
* Permissions of this copyleft license are conditioned on making available
* complete source code of licensed works and modifications under the same
* license or the GNU GPLv3. Copyright and license notices must be preserved.
* Contributors provide an express grant of patent rights. However, a larger
* work using the licensed work through interfaces provided by the licensed
* work may be distributed under different terms and without source code for
* the larger work.
*
* Copyright (C) 2015 Jean Machuca,
*
* Everyone is permitted to copy and distribute verbatim copies of this
* license document, but changing it is not allowed.
*/
Package('cl.quickcorp.components',[
Class('FormField',Component,{
cached:false,
reload:true,
createBindingEvents:function (){
var _executeBinding = this.executeBinding;
var thisobj = this;
var _objList = this.body.querySelectorAll(this.fieldType);
for (var _datak=0;_datak<_objList.length;_datak++){
var _obj = _objList[_datak];
_obj.addEventListener('change',function(e){
logger.debug('Executing change event binding');
thisobj.executeBindings();
});
_obj.addEventListener('keydown',function(e){
logger.debug('Executing keydown event binding');
thisobj.executeBindings();
});
}
},
executeBinding:function (_obj){
var _datamodel = _obj.getAttribute('data-field');
logger.debug('Binding '+_datamodel+' for '+this.name);
this.data[_datamodel]=_obj.value;
},
executeBindings:function (){
var _objList = this.body.querySelectorAll(this.fieldType);
for (var _datak=0;_datak<_objList.length;_datak++){
var _obj = _objList[_datak];
var _datamodel = _obj.getAttribute('data-field');
logger.debug('Binding '+_datamodel+' for '+this.name);
this.data[_datamodel]=_obj.value;
}
},
done:function (){
var thisobj = this;
thisobj.executeBindings();
thisobj.createBindingEvents();
logger.debug('Field loaded: '+thisobj.fieldType+'[name='+thisobj.name+']');
}
}),
Class('ButtonField',FormField,{
fieldType:'button'
}),
Class('InputField',FormField,{
fieldType:'input'
}),
Class('TextField',FormField,{
fieldType:'textarea'
}),
Class('EmailField',FormField,{
fieldType:'input'
})
]);
```
## Step 4: Once you have done the above components declaration, you will now want to code your controllers (cl.quickcorp.controller.js)
```javascript
"use strict";
/*
* QuickCorp/QCObjects is licensed under the
* GNU Lesser General Public License v3.0
* [LICENSE] (https://github.com/QuickCorp/QCObjects/blob/master/LICENSE.txt)
*
* Permissions of this copyleft license are conditioned on making available
* complete source code of licensed works and modifications under the same
* license or the GNU GPLv3. Copyright and license notices must be preserved.
* Contributors provide an express grant of patent rights. However, a larger
* work using the licensed work through interfaces provided by the licensed
* work may be distributed under different terms and without source code for
* the larger work.
*
* Copyright (C) 2015 Jean Machuca,
*
* Everyone is permitted to copy and distribute verbatim copies of this
* license document, but changing it is not allowed.
*/
"use strict";
Package('cl.quickcorp.controller',[
Class('MainController',Object,{
_new_:function (){
//TODO: Implement
logger.debug('MainController Element Initialized');
}
}),
Class('MyAccountController',Object,{
component: null,
done:function (){
var controller = this;
logger.debug('MyAccountController Element Initialized');
this.component.body.setAttribute('loaded',true);
},
_new_:function (o){
//TODO: Implement
this.component = o.component;
}
}),
]);
```
## Step 5: To use into the HTML5 code you only need to do some settings between
```
View on NPM
View on GitHub
QCObjects version 1.0.0
### Dependencies
QCObjects version 1.4.2
### Dependencies
QCObjects version 2.1.2
### Dependencies
QCObjects version 2.1.3
### Dependencies
QCObjects version 2.1.4
### Dependencies
QCObjects version 2.1.5
### Dependencies
QCObjects version 2.1.6
### Dependencies
QCObjects version 2.1.10
### Dependencies
QCObjects version 2.1.11
### Dependencies
QCObjects version 2.1.12
### Dependencies
QCObjects version 2.1.13
### Dependencies
QCObjects version 2.1.7
### Dependencies
QCObjects version 2.1.8
### Dependencies
QCObjects version 2.1.9
### Dependencies
QCObjects version 2.1.14
### Dependencies
QCObjects version 2.1.15
### Dependencies
QCObjects version 2.1.16
### Dependencies
QCObjects version 2.1.17
### Dependencies
QCObjects version 2.1.171
### Dependencies
QCObjects version 2.1.172
### Dependencies
QCObjects version 2.1.173
### Dependencies
QCObjects version 2.1.174
### Dependencies
QCObjects version 2.1.175
### Dependencies
QCObjects version 2.1.176
### Dependencies
QCObjects version 2.1.177
### Dependencies
QCObjects version 2.1.178
### Dependencies
QCObjects version 2.1.179
### Dependencies
QCObjects version 2.1.180
### Dependencies
QCObjects version 2.1.181
### Dependencies
QCObjects version 2.1.182
### Dependencies
QCObjects version 2.1.183
### Dependencies
QCObjects version 2.1.184
### Dependencies
QCObjects version 2.1.185
### Dependencies
QCObjects version 2.1.186
### Dependencies
QCObjects version 2.1.187
### Dependencies
QCObjects version 2.1.188
### Dependencies
QCObjects version 2.1.189
### Dependencies
QCObjects version 2.1.190
### Dependencies
QCObjects version 2.1.191
### Dependencies
QCObjects version 2.1.192
### Dependencies
QCObjects version 2.1.193
### Dependencies
QCObjects version 2.1.194
### Dependencies
QCObjects version 2.1.195
### Dependencies
QCObjects version 2.1.196
### Dependencies
QCObjects version 2.1.197
### Dependencies
QCObjects version 2.1.198
### Dependencies
QCObjects version 2.1.199
### Dependencies
QCObjects version 2.1.200
### Dependencies
QCObjects version 2.1.201
### Dependencies
QCObjects version 2.1.202
### Dependencies
QCObjects version 2.1.203
### Dependencies
QCObjects version 2.1.204
### Dependencies
QCObjects version 2.1.205
### Dependencies
QCObjects version 2.1.206
### Dependencies